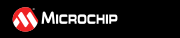 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
14 #include "config/hal.h"
15 #include "app_power_control.h"
17 #include "devices/dev_buck_converter.h"
18 #include "drivers/v_loop.h"
34 volatile uint16_t retval = 1;
245 volatile uint16_t retval = 1;
387 volatile uint16_t retval=1;
volatile uint16_t ExtHookPreAntiWindupFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
volatile bool async_mode
Bit #9: Control bit suspending the synchronous rectifier switch PWM channel.
volatile uint16_t period
Soft-Start Period (POD, RAMP PERIOD, PGD, etc.)
volatile uint16_t * ptrADCTriggerARegister
Pointer to ADC trigger #1 register (e.g. TRIG1)
volatile bool differential_input
input channel differential mode enable bit
volatile bool interrupt_enable
input channel interrupt enable bit
volatile uint16_t ptrExtHookStopFunction
Pointer to Function which is called at the end of the control loop but will be bypassed when the cont...
volatile int16_t AltMaxOutput
Alternate maximum output value used for clamping (R/W)
#define BUCK_PWM_OUTPUT_SWAP
true = PWMxH is the leading PWM output, false = PWMxL is the leading PWM output
#define BUCK_ISNS_ADCIN
Analog input number (e.g. '5' for 'AN5')
volatile struct NPNZ16b_s v_loop
External reference to user-defined NPNZ16b controller data object 'v_loop'.
volatile uint16_t gpio_high
GPIO port pin-number of PWMxH of the selected PWM generator.
volatile uint16_t ADCTriggerBOffset
ADC trigger #2 offset to compensate propagation delays.
#define BUCK_VOUT_ADCTRIG
Register used for trigger placement.
volatile bool swap_source
Bit 13: when set, AltSource is used as data input to controller.
volatile uint16_t dead_time_rising
Dead time setting at rising edge of a half-bridge drive.
volatile uint16_t ExtHookSourceFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
volatile bool invert_input
Bit 14: when set, most recent error input value to controller is inverted.
volatile uint16_t AgcScaler
Bit-shift scaler of Adaptive Gain Modulation factor.
#define PWM_CLOCK_HIGH_RESOLUTION
Enables/disables the PWM generator high resolution mode of 250 ps versus 2 ns.
#define BUCK_PWM_PDC
PWM Instance Duty Cycle Register.
volatile uint16_t reference
Control loop reference variable.
volatile bool swap_outputs
Selecting if PWMxH (default) or PWMxL should be the leading PWM output.
volatile struct BUCK_CONVERTER_STARTUP_s startup
BUCK startup timing settings.
volatile uint16_t ExtHookTargetFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
void(* ctrl_Update)(volatile struct NPNZ16b_s *)
Function pointer to UPDATE routine.
volatile uint16_t counter
Soft-Start Execution Counter (read only)
volatile uint16_t * ptrDProvControlInputCompensated
Pointer to external data buffer of most recent, compensated control input.
#define BUCK_PWM_GPIO_PORT_PINH
Port Pin Number.
volatile uint16_t usrParam7
generic 16-bit wide, user-defined parameter #8 for advanced control options
void v_loop_Precharge(volatile struct NPNZ16b_s *controller, volatile fractional ctrl_input, volatile fractional ctrl_output)
Prototype of the Assembly routine '_v_loop_Precharge' loading user-defined values into the NPNZ16b co...
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s v_ramp
volatile struct BUCK_FEEDBACK_SETTINGS_s feedback
BUCK converter feedback settings.
volatile int16_t factor
Fractional scaling factor (range -1 ... 0.99969)
#define BUCK_ISNS_REF
Output Current Reference.
volatile uint16_t trigger_scaler
PWM triggers for ADC will be generated every n-th cycle.
enum BUCK_CONTROL_MODE_e control_mode
Fundamental control mode.
volatile uint16_t v_in
BUCK input voltage.
#define PWRGOOD_PIN
GPIO port pin declaration where 0=Rx0, 1=Rx1, 2=Rx3, etc.
volatile uint16_t io_type
Input/Output definition (0=push-pull output, 1=input, 2=open-drain output)
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
volatile int16_t Offset
Value/signal offset of this port.
volatile uint16_t appPowerSupply_ConverterObjectInitialize(void)
This function initializes the buck converter device driver instance.
#define BUCK_VIN_OFFSET
Input voltage feedback offset.
volatile uint8_t adc_core
number of the ADC core connected to the selected channel
volatile uint16_t gpio_instance
GPIO instance of the selected PWM generator.
volatile bool lower_saturation_event
Bit 0: control loop is clamped at minimum output level.
volatile uint16_t gpio_low
GPIO port pin-number of PWMxL of the selected PWM generator.
volatile uint16_t appPowerSupply_ControllerInitialize(void)
This function initializes the control system feedback loop objects.
volatile uint8_t trigger_source
input channel trigger source
volatile struct NPNZ_DATA_PROVIDERS_s DataProviders
Automated data sources pushing recent data points to user-defined variables.
volatile uint16_t duty_ratio_min
Absolute duty cycle minimum during normal operation.
#define BUCK_ISNS_ADCTRIG
Register used for trigger placement.
volatile uint16_t control_input
BUCK most recent control input value (raw input)
#define BUCK_PWM_ADTR1OFS
ADC Trigger 1 Offset: 0...31.
volatile uint16_t i_sns[BUCK_MPHASE_COUNT]
BUCK output current.
#define BUCK_VIN_ADCCORE
0=Dedicated Core #0, 1=Dedicated Core #1, 8=Shared ADC Core
volatile uint16_t usrParam4
generic 16-bit wide, user-defined parameter #5 for advanced control options
volatile struct NPNZ_PORT_s Target
Primary data output port declaration.
volatile struct NPNZ_ADC_TRGCTRL_s ADCTriggerControl
Automatic ADC trigger placement options for ADC Trigger A and B.
volatile bool fault_active
Bit #5: Flag bit indicating system is in enforced shut down mode (usually due to a fault condition)
#define BUCK_ISNS_FB_OFFSET
volatile bool enabled
input channel enable bit
#define PWRGOOD_PORT
GPIO port declaration where 0=Port RA, 0=Port RB, 0=Port RC, etc.
volatile uint16_t usrParam1
generic 16-bit wide, user-defined parameter #2 for advanced control options
volatile uint16_t ptrExtHookEndFunction
Pointer to Function which is called at the end of the control loop and will also be called when the c...
volatile bool sync_drive
Selecting if switch node is driven in synchronous or asnchronous mode.
volatile uint16_t reference
Internal dummy reference used to increment/decrement controller reference.
volatile uint16_t polarity
Output polarity, where 0=ACTIVE HIGH, 1=ACTIVE_LOW.
volatile struct NPNZ_PORT_s AltTarget
Secondary data output port declaration.
volatile uint16_t ADCTriggerAOffset
ADC trigger #1 offset to compensate propagation delays.
#define BUCK_PWM_DEAD_TIME_LE
Rising edge dead time [tick = 250ps].
volatile uint16_t minimum
output clamping value (minimum)
volatile struct NPNZ_STATUS_s status
Control Loop Status and Control flags.
volatile int16_t MaxOutput
Maximum output value used for clamping (R/W)
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vout
ADC input sampling output voltage.
volatile uint8_t adc_input
number of the ADC input channel used
volatile uint16_t ptrExtHookPreAntiWindupFunction
Pointer to Function which will be called after the compensation filter computation is complete and be...
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s power_on_delay
volatile bool level_trigger
input channel level trigger mode enable bit
volatile uint16_t drv_BuckConverter_Initialize(volatile struct BUCK_CONVERTER_s *buckInstance)
This function initializes all peripheral modules and their instances used by the power controller.
#define BUCK_VOUT_NORM_SCALER
VOUT normalization bit-shift scaler
volatile uint16_t * ptrDProvControlInput
Pointer to external data buffer of most recent, raw control input.
volatile uint16_t pwm_instance
number of the PWM channel used
#define BUCK_PWM_CHANNEL
PWM peripheral output pins, control signals and register assignments of converter phase #1.
#define BUCK_PWM_DEAD_TIME_FE
Falling edge dead time [tick = 250ps].
#define BUCK_ISNS_ADCBUF
ADC input buffer of this ADC channel.
#define BUCK_PWM_DC_MIN
This sets the minimum duty cycle.
volatile uint16_t * ptrDProvControlOutput
Pointer to external data buffer of most recent control output.
#define BUCK_VOUT_ADCBUF
ADC input buffer of this ADC channel.
#define BUCK_PWM_PERIOD
This sets the switching period of the converter.
#define BUCK_VIN_TRGSRC
PWM1 (=PG1) Trigger 2 via PGxTRIGB.
#define BUCK_ISNS_ADCCORE
0=Dedicated Core #0, 1=Dedicated Core #1, 2=Shared ADC Core
volatile int16_t MinOutput
Minimum output value used for clamping (R/W)
volatile uint16_t v_loop_Initialize(volatile struct NPNZ16b_s *controller)
Initializes controller coefficient arrays and normalization factors.
#define BUCK_VOUT_REF
Macro calculating the integer number equivalent of the output voltage reference given above in [V].
volatile bool upper_saturation_event
Bit 1: control loop is clamped at maximum output level.
volatile bool enabled
Specifies, if this IO is used or not.
volatile bool enabled
Bit 15: enables/disables control loop execution.
volatile bool ready
Bit #0: status bit, indicating buck converter is initialized and ready to run.
volatile struct NPNZ_EXTENSION_HOOKS_s ExtensionHooks
User extension function triggers using function pointers with parameters.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vin
ADC input sampling input voltage.
volatile uint16_t appPowerSupply_PeripheralsInitialize(void)
This function is used to load peripheral configuration templates from the power controller device dri...
void v_loop_Reset(volatile struct NPNZ16b_s *controller)
Prototype of the Assembly routine '_v_loop_Reset' clearing the NPNZ16b controller output and error hi...
volatile uint16_t phase
Switching signal phase-shift.
volatile uint16_t duty_ratio_init
Initial duty cycle when the PWM module is being turned on.
#define BUCK_NO_OF_PHASES
User-declaration of global defines for PWM signal generator settings.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s i_ramp
volatile uint16_t trigger_offset
PWM triggers for ADC will be offset by n cycles.
volatile uint16_t ptrExtHookStartFunction
Pointer to Function which will be called at the beginning of the control loop.
volatile int16_t NormScaler
Bit-shift scaler of the Q15 normalization factor.
volatile struct BUCK_SWITCH_NODE_SETTINGS_s sw_node[BUCK_MPHASE_COUNT]
BUCK converter switch node settings.
#define BUCK_VIN_ADCBUF
ADC input buffer of this ADC channel.
#define BUCK_PWM_GPIO_INSTANCE
Number indicating device port, where 0=Port RA, 0=Port RB, 0=Port RC, etc.
volatile struct BUCK_GPIO_INSTANCE_s EnableInput
External ENABLE input.
volatile uint16_t port
GPIO port instance number (0=Port RA, 0=Port RB, 0=Port RC, etc.)
volatile uint16_t ptrExtHookTargetFunction
Pointer to Function which will be called before the most recent control output is written to target.
volatile uint16_t(* ctrl_Initialize)(volatile struct NPNZ16b_s *)
Function pointer to INIT routine.
#define BUCK_VIN_ADCIN
Analog input number (e.g. '5' for 'AN5')
volatile uint16_t control_output
BUCK most recent control output value.
volatile fractional NormFactor
Q15 normalization factor.
volatile struct BUCK_CONVERTER_DATA_s data
BUCK runtime data.
#define BUCK_VIN_ANSEL
GPIO analog function mode enable bit.
void(* ctrl_Reset)(volatile struct NPNZ16b_s *)
Function pointer to RESET routine.
volatile int16_t offset
Signal offset as signed integer to be subtracted from ADC input.
volatile struct BUCK_GPIO_SETTINGS_s gpio
BUCK converter additional GPIO specification.
volatile struct NPNZ_PORT_s AltSource
Secondary data input port declaration.
volatile uint16_t trigger_offset
ADC trigger offset value for trigger fine-tuning.
volatile bool swap_target
Bit 12: when set, AltTarget is used as data output of controller.
volatile uint16_t maximum
output clamping value (maximum)
void(* ctrl_Precharge)(volatile struct NPNZ16b_s *, volatile fractional, volatile fractional)
Function pointer to PRECHARGE routine.
volatile uint16_t ExtHookStopFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
#define BUCK_ISNS_OFFSET_CALIBRATION_ENABLE
Current Sense Offset Calibration is disabled.
volatile uint16_t usrParam6
generic 16-bit wide, user-defined parameter #7 for advanced control options
#define BUCK_VIN_NORM_FACTOR
VIN normalization factor scaled in Q15.
#define BUCK_ISNS_NORM_FACTOR
ISNS normalization factor scaled in Q15.
#define BUCK_VOUT_ANSEL
ADC input assignments of output voltage feedback signals.
volatile uint16_t ExtHookStartFunctionParam
Parameter of function called (can be a variable or pointer to a data structure)
#define BUCK_LEB_PERIOD
Leading Edge Blanking = n x PWM resolution (here: 50 x 2ns = 100ns)
volatile uint16_t v_ref
User reference setting used to control the power converter controller.
void v_loop_Update(volatile struct NPNZ16b_s *controller)
Prototype of the Assembly feedback control loop routine helping to call the v_loop controller from C-...
volatile uint16_t usrParam0
generic 16-bit wide, user-defined parameter #1 for advanced control options
volatile bool cs_calib_enable
Bit #8: Flag bit indicating that current sensors need to calibrated.
volatile int16_t scaler
Feedback bit-shift scaler used for number normalization.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_isns[BUCK_MPHASE_COUNT]
ADC input sampling phase current.
#define TEMP_ANSEL
GPIO analog function mode enable bit.
volatile uint16_t ref_inc_step
Size/value of one reference increment/decrement or this period.
volatile struct BUCK_CONVERTER_SETTINGS_s set_values
Control field for global access to references.
volatile uint16_t pin
GPIO port pin number.
#define BUCK_ISNS_ADC_TRGDLY
volatile bool agc_enabled
Bit 11: when set, Adaptive Gain Control Modulation is enabled.
volatile struct BUCK_GPIO_INSTANCE_s PowerGood
Power Good Output.
volatile bool pwm_active
Bit #2: indicating that PWM has been started and ADC triggers are generated.
volatile bool master_period_enable
Selecting MASTER or Individual period register.
volatile uint16_t leb_period
Leading-Edge Blanking period.
volatile uint16_t ptrExtHookSourceFunction
Pointer to Function which will be called after the source has been read and compensated.
#define BUCK_PWM_DC_MAX
This sets the maximum duty cycle.
volatile uint16_t duty_ratio_max
Absolute duty cycle maximum during normal operation.
volatile bool early_interrupt_enable
input channel early interrupt enable bit
#define BUCK_VOUT_NORM_FACTOR
VOUT normalization factor scaled in Q15.
volatile uint16_t usrParam2
generic 16-bit wide, user-defined parameter #3 for advanced control options
volatile uint16_t i_ref
User reference setting used to control the power converter controller.
#define BUCK_PWM_PHASE_SHIFT
This sets the phase shift between phase #1 and #2.
volatile struct NPNZ_PORT_s Source
Primary data input port declaration.
#define BUCK_ISNS_NORM_SCALER
ISNS normalization
volatile struct NPNZ16b_s * controller
pointer to control loop object data structure
volatile struct NPNZ_LIMITS_s Limits
Input and output clamping values.
volatile uint16_t temp
BUCK board temperature.
volatile bool adc_active
Bit #1: indicating that ADC has been started and samples are taken.
volatile uint16_t ExtHookEndFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
#define BUCK_VOUT_ADCCORE
0=Dedicated Core #0, 1=Dedicated Core #1, 8=Shared ADC Core
volatile struct BUCK_STATE_ID_s state_id
BUCK state machine operating state ID.
volatile uint16_t dead_time_falling
Dead time setting at falling edge of a half-bridge drive.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s power_good_delay
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_temp
ADC input sampling temperature.
volatile uint16_t * ptrDProvControlError
Pointer to external data buffer of most recent control error.
volatile fractional AgcFactor
Q15 value of Adaptive Gain Modulation factor.
#define BUCK_PWM_ADTR1PS
ADC Trigger 1 Postscaler: 0...31.
volatile uint16_t * ptrADCTriggerBRegister
Pointer to ADC trigger #2 register (e.g. TRIG2)
volatile struct BUCK_LOOP_SETTINGS_s v_loop
BUCK voltage control loop object.
#define BUCK_VOUT_OFFSET
Macro calculating the integer number equivalent of the physical, static signal offset of this feedbac...
#define BUCK_PWM_GPIO_PORT_PINL
Port Pin Number.
volatile uint16_t control_error
BUCK most recent control error value.
#define BUCK_VOUT_TRGSRC
PWM1 (=PG1) Trigger 1 via PGxTRIGA.
#define BUCK_VIN_NORM_SCALER
VIN normalization
#define TEMP_ADCBUF
GPIO analog function mode enable bit.
volatile struct NPNZ_PORTS_s Ports
Controller input and output ports.
volatile struct BUCK_ADC_INPUT_SCALING_s scaling
normalization scaling settings
#define BUCK_ISNS_TRGSRC
PWM1 (=PG1) Trigger 2 via PGxTRIGB.
volatile uint16_t no_of_phases
number of converter phases
volatile struct NPNZ_GAIN_CONTROL_s GainControl
Parameter section for advanced control options.
volatile uint16_t usrParam3
generic 16-bit wide, user-defined parameter #4 for advanced control options
volatile uint16_t period
Switching period.
volatile bool signed_result
input channel singed result mode enable bit
#define BUCK_VOUT_ADC_TRGDLY
Macro calculating the integer number equivalent of the signal chain time delay between internal PWM t...
volatile struct BUCK_CONVERTER_s buck
Global data object for a BUCK CONVERTER.
volatile fractional AgcMedian
Q15 value of Adaptive Gain Modulation nominal operating point.
#define BUCK_ISNS_ANSEL
GPIO analog function mode enable bit.
volatile struct NPNZ_USER_DATA_BUFFER_s Advanced
Parameter section for advanced user control options.
volatile int16_t AltMinOutput
Alternate minimum output value used for clamping (R/W)
volatile uint16_t feedback_offset
Feedback offset value for calibration or bi-direction feedback signals.
volatile uint16_t * ptrAddress
Pointer to register or variable where the value is read from (e.g. ADCBUFx) or written to (e....
volatile bool high_resolution_enable
Selecting if PWM module should use high-resolution mode.
#define BUCK_POD
Conversion Macros of Startup Timing Settings.
volatile bool enabled
Bit #15: Control bit enabling/disabling the charger port.
volatile uint16_t v_out
BUCK output voltage.
volatile uint16_t usrParam5
generic 16-bit wide, user-defined parameter #6 for advanced control options
#define BUCK_VOUT_ADCIN
Analog input number (e.g. '5' for 'AN5')
volatile bool autorun
Bit #14: Control bit determining if charger is starting automatically or on command (using the GO bit...
volatile uint16_t * adc_buffer
pointer to ADC result buffer
volatile uint16_t * ptrControlReference
Pointer to global variable of input register holding the controller reference value (e....