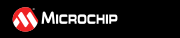 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
45 #ifndef BUCK_CONVERTER_TYPE_DEF_H
46 #define BUCK_CONVERTER_TYPE_DEF_H
53 #include "power_control/drivers/npnz16b.h"
54 #include "config/hal.h"
62 #define BUCK_MPHASE_COUNT BUCK_NO_OF_PHASES
73 BUCK_STAT_READY = 0b0000000000000001,
74 BUCK_STAT_ADC_ACTIVE = 0b0000000000000010,
75 BUCK_STAT_PWM_ACTIVE = 0b0000000000000100,
76 BUCK_STAT_POWERSOURCE_DETECTED = 0b0000000000001000,
77 BUCK_STAT_CS_SENSE_READY = 0b0000000000010000,
79 BUCK_STAT_FORCED_SHUT_DOWN = 0b0000000010000000,
80 BUCK_STAT_BUSY = 0b0000000100000000,
83 BUCK_STAT_GO = 0b0010000000000000,
84 BUCK_STAT_AUTORUN = 0b0100000000000000,
85 BUCK_STAT_NO_AUTORUN = 0b0000000000000000,
87 BUCK_STAT_ENABLED = 0b1000000000000000,
88 BUCK_STAT_DISABLED = 0b0000000000000000
101 BUCK_OPSTATE_ERROR = 0x00,
102 BUCK_OPSTATE_INITIALIZE = 0x01,
103 BUCK_OPSTATE_RESET = 0x02,
104 BUCK_OPSTATE_STANDBY = 0x03,
105 BUCK_OPSTATE_RAMPUP = 0x04,
106 BUCK_OPSTATE_ONLINE = 0x05
120 BUCK_OPSTATE_POWER_ON_DELAY = 0x00,
121 BUCK_OPSTATE_PREPARE_V_RAMP = 0x01,
122 BUCK_OPSTATE_V_RAMP_UP = 0x02,
123 BUCK_OPSTATE_I_RAMP_UP = 0x03,
124 BUCK_OPSTATE_PWRGOOD_DELAY = 0x04
137 BUCK_OPSRET_ERROR = 0x0000,
138 BUCK_OPSRET_COMPLETE = 0x0001,
139 BUCK_OPSRET_REPEAT = 0x0002
153 BUCK_CONTROL_MODE_VMC = 0,
155 BUCK_CONTROL_MODE_ACMC = 2
225 } __attribute__((packed)) bits;
volatile bool async_mode
Bit #9: Control bit suspending the synchronous rectifier switch PWM channel.
volatile uint16_t period
Soft-Start Period (POD, RAMP PERIOD, PGD, etc.)
volatile bool differential_input
input channel differential mode enable bit
volatile bool interrupt_enable
input channel interrupt enable bit
volatile uint16_t gpio_high
GPIO port pin-number of PWMxH of the selected PWM generator.
volatile uint16_t dead_time_rising
Dead time setting at rising edge of a half-bridge drive.
volatile uint16_t reference
Control loop reference variable.
volatile bool swap_outputs
Selecting if PWMxH (default) or PWMxL should be the leading PWM output.
volatile struct BUCK_CONVERTER_STARTUP_s startup
BUCK startup timing settings.
void(* ctrl_Update)(volatile struct NPNZ16b_s *)
Function pointer to UPDATE routine.
volatile uint16_t counter
Soft-Start Execution Counter (read only)
enum BUCK_SUBSTATES_e SubStateOpCodes
List of State Machine Sub-State IDs.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s v_ramp
volatile struct BUCK_FEEDBACK_SETTINGS_s feedback
BUCK converter feedback settings.
volatile int16_t factor
Fractional scaling factor (range -1 ... 0.99969)
Buck converter feedback declarations.
volatile struct BUCK_CONVERTER_CONSTANTS_s BuckConverterConstants
Structure providing all public enumerated lists of constants.
volatile uint16_t trigger_scaler
PWM triggers for ADC will be generated every n-th cycle.
enum BUCK_CONTROL_MODE_e control_mode
Fundamental control mode.
volatile uint16_t v_in
BUCK input voltage.
enum BUCK_STATUS_FLAGS_e StatusFlags
List of all status and control flags of the Buck Converter status word.
User defined settings for control loops;.
BUCK_CONTROL_MODE_e
Enumeration of the power supply mode control.
volatile uint16_t io_type
Input/Output definition (0=push-pull output, 1=input, 2=open-drain output)
volatile struct BUCK_LOOP_SETTINGS_s i_loop[BUCK_MPHASE_COUNT]
BUCK Current control loop objects.
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
Global NPNZ controller data object.
Power Converter Startup Timing Settings.
volatile uint8_t adc_core
number of the ADC core connected to the selected channel
volatile uint16_t gpio_instance
GPIO instance of the selected PWM generator.
volatile uint16_t gpio_low
GPIO port pin-number of PWMxL of the selected PWM generator.
volatile uint8_t trigger_source
input channel trigger source
volatile uint16_t duty_ratio_min
Absolute duty cycle minimum during normal operation.
volatile uint16_t control_input
BUCK most recent control input value (raw input)
GPIO instance of the converter control GPIO.
ADC input channel configuration.
volatile uint16_t i_sns[BUCK_MPHASE_COUNT]
BUCK output current.
volatile bool busy
Bit #7: Flag bit indicating that the state machine is executing a process (e.g. startup-ramp)
volatile bool fault_active
Bit #5: Flag bit indicating system is in enforced shut down mode (usually due to a fault condition)
volatile bool enabled
input channel enable bit
volatile bool sync_drive
Selecting if switch node is driven in synchronous or asnchronous mode.
volatile uint16_t reference
Internal dummy reference used to increment/decrement controller reference.
volatile uint16_t polarity
Output polarity, where 0=ACTIVE HIGH, 1=ACTIVE_LOW.
volatile unsigned
Bit #3: (reserved)
volatile uint16_t minimum
output clamping value (minimum)
volatile bool suspend
Bit #6: Control bit to put the converter in suspend mode (turned off while ENABLE bit is still on)
Generic power controller status word.
enum BUCK_OPSTATES_e opstate_id
Most recent operating state of main state machine.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vout
ADC input sampling output voltage.
volatile uint8_t adc_input
number of the ADC input channel used
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s power_on_delay
volatile bool level_trigger
input channel level trigger mode enable bit
volatile uint16_t pwm_instance
number of the PWM channel used
enum BUCK_OPSTATES_e OpStateOpCodes
List of State Machine Operating State IDs.
volatile bool enabled
Specifies, if this IO is used or not.
BUCK_SUBSTATES_e
Enumeration of state machine operating sub-states.
volatile bool ready
Bit #0: status bit, indicating buck converter is initialized and ready to run.
enum BUCK_SUBSTATES_e substate_id
Most recent operating state of active sub state machine.
Generic power controller control settings.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vin
ADC input sampling input voltage.
volatile uint16_t phase
Switching signal phase-shift.
volatile uint16_t duty_ratio_init
Initial duty cycle when the PWM module is being turned on.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s i_ramp
enum BUCK_OPSTATE_RETURNS_e OpStateReturnValues
List of State Machine Operating State Return Values.
volatile uint16_t trigger_offset
PWM triggers for ADC will be offset by n cycles.
volatile struct BUCK_SWITCH_NODE_SETTINGS_s sw_node[BUCK_MPHASE_COUNT]
BUCK converter switch node settings.
#define BUCK_MPHASE_COUNT
Declaration of the number of power train phases of the Buck Converter.
volatile struct BUCK_GPIO_INSTANCE_s EnableInput
External ENABLE input.
volatile uint16_t port
GPIO port instance number (0=Port RA, 0=Port RB, 0=Port RC, etc.)
volatile uint16_t(* ctrl_Initialize)(volatile struct NPNZ16b_s *)
Function pointer to INIT routine.
volatile uint16_t control_output
BUCK most recent control output value.
volatile struct BUCK_CONVERTER_DATA_s data
BUCK runtime data.
void(* ctrl_Reset)(volatile struct NPNZ16b_s *)
Function pointer to RESET routine.
volatile int16_t offset
Signal offset as signed integer to be subtracted from ADC input.
volatile struct BUCK_GPIO_SETTINGS_s gpio
BUCK converter additional GPIO specification.
volatile uint16_t trigger_offset
ADC trigger offset value for trigger fine-tuning.
volatile uint16_t maximum
output clamping value (maximum)
Switching signal timing settings.
void(* ctrl_Precharge)(volatile struct NPNZ16b_s *, volatile fractional, volatile fractional)
Function pointer to PRECHARGE routine.
Publicly accessible data buffer of most recent runtime data values.
volatile uint16_t v_ref
User reference setting used to control the power converter controller.
volatile bool cs_calib_enable
Bit #8: Flag bit indicating that current sensors need to calibrated.
volatile int16_t scaler
Feedback bit-shift scaler used for number normalization.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_isns[BUCK_MPHASE_COUNT]
ADC input sampling phase current.
volatile uint16_t ref_inc_step
Size/value of one reference increment/decrement or this period.
volatile struct BUCK_CONVERTER_SETTINGS_s set_values
Control field for global access to references.
volatile uint16_t pin
GPIO port pin number.
volatile bool cs_calib_complete
Bit #4: indicating that current sensor calibration has completed.
volatile uint16_t i_out
BUCK common output current.
volatile struct BUCK_GPIO_INSTANCE_s PowerGood
Power Good Output.
volatile bool pwm_active
Bit #2: indicating that PWM has been started and ADC triggers are generated.
volatile bool master_period_enable
Selecting MASTER or Individual period register.
Generic power controller startup period settings.
volatile uint16_t leb_period
Leading-Edge Blanking period.
BUCK_OPSTATES_e
Enumeration of state machine operating states.
data structure for the buck statement ID for sub-operating and operating states
volatile uint16_t duty_ratio_max
Absolute duty cycle maximum during normal operation.
volatile bool early_interrupt_enable
input channel early interrupt enable bit
volatile uint16_t i_ref
User reference setting used to control the power converter controller.
volatile struct NPNZ16b_s * controller
pointer to control loop object data structure
volatile uint16_t temp
BUCK board temperature.
volatile bool adc_active
Bit #1: indicating that ADC has been started and samples are taken.
BUCK control & monitoring data structure.
struct BUCK_STATE_ID_s::@3::@4 bits
volatile struct BUCK_STATE_ID_s state_id
BUCK state machine operating state ID.
volatile uint16_t dead_time_falling
Dead time setting at falling edge of a half-bridge drive.
volatile uint16_t gpio_instance
GPIO instance of the selected PWM generator.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s power_good_delay
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_temp
ADC input sampling temperature.
volatile struct BUCK_LOOP_SETTINGS_s v_loop
BUCK voltage control loop object.
BUCK_STATUS_FLAGS_e
Enumeration of status and control flags.
volatile uint16_t control_error
BUCK most recent control error value.
GPIO instance of the converter control GPIO.
ADC input signal scaling = (ADCBUF - <offset>) * <factor> >> 2^<scaler>
volatile bool GO
Bit #13: When set, the GO-bit fires up the power supply.
volatile struct BUCK_ADC_INPUT_SCALING_s scaling
normalization scaling settings
volatile uint16_t no_of_phases
number of converter phases
volatile uint16_t period
Switching period.
volatile bool signed_result
input channel singed result mode enable bit
Structure providing all public enumerated lists of constants.
volatile uint16_t feedback_offset
Feedback offset value for calibration or bi-direction feedback signals.
volatile bool high_resolution_enable
Selecting if PWM module should use high-resolution mode.
enum BUCK_CONTROL_MODE_e ControlModes
List of Supported Control Modes.
volatile bool enabled
Bit #15: Control bit enabling/disabling the charger port.
volatile uint16_t v_out
BUCK output voltage.
BUCK_OPSTATE_RETURNS_e
Enumeration of state machine operating state return values.
volatile bool autorun
Bit #14: Control bit determining if charger is starting automatically or on command (using the GO bit...
volatile uint16_t * adc_buffer
pointer to ADC result buffer