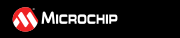 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
42 volatile uint16_t v_loop_ACoefficients_size = (
sizeof(v_loop_coefficients.ACoefficients)/
sizeof(v_loop_coefficients.ACoefficients[0]));
43 volatile uint16_t v_loop_BCoefficients_size = (
sizeof(v_loop_coefficients.BCoefficients)/
sizeof(v_loop_coefficients.BCoefficients[0]));
46 volatile uint16_t v_loop_ControlHistory_size = (
sizeof(v_loop_histories.ControlHistory)/
sizeof(v_loop_histories.ControlHistory[0]));
47 volatile uint16_t v_loop_ErrorHistory_size = (
sizeof(v_loop_histories.ErrorHistory)/
sizeof(v_loop_histories.ErrorHistory[0]));
65 volatile int32_t v_loop_ACoefficients [4] =
73 volatile int32_t v_loop_BCoefficients [5] =
83 volatile int16_t v_loop_pre_scaler = 3;
84 volatile int16_t v_loop_post_shift_A = 0;
85 volatile int16_t v_loop_post_shift_B = 0;
86 volatile fractional v_loop_post_scaler = 0x0000;
109 volatile uint16_t i=0;
131 v_loop_coefficients.ACoefficients[i] = v_loop_ACoefficients[i];
132 v_loop_coefficients.ACoefficients[i] = v_loop_ACoefficients[i];
133 v_loop_coefficients.ACoefficients[i] = v_loop_ACoefficients[i];
134 v_loop_coefficients.ACoefficients[i] = v_loop_ACoefficients[i];
140 v_loop_coefficients.BCoefficients[i] = v_loop_BCoefficients[i];
141 v_loop_coefficients.BCoefficients[i] = v_loop_BCoefficients[i];
142 v_loop_coefficients.BCoefficients[i] = v_loop_BCoefficients[i];
143 v_loop_coefficients.BCoefficients[i] = v_loop_BCoefficients[i];
144 v_loop_coefficients.BCoefficients[i] = v_loop_BCoefficients[i];
volatile struct NPNZ16b_s v_loop
External reference to user-defined NPNZ16b controller data object 'v_loop'.
volatile int32_t * ptrACoefficients
Pointer to A coefficients located in X-space.
Global NPNZ controller data object.
volatile uint16_t ControlHistoryArraySize
Size of the control history array in Y-space.
volatile uint16_t value
Controller status full register access.
volatile fractional * ptrErrorHistory
Pointer to n+1 delay-line samples located in Y-space with first sample being the most recent.
volatile struct NPNZ_FILTER_PARAMS_s Filter
Filter parameters such as pointer to history and coefficient arrays and number scaling.
volatile struct NPNZ_STATUS_s status
Control Loop Status and Control flags.
volatile int16_t v_loop_pterm_scaler
Bit-shift scaler of the P-Term Coefficient for Plant Measurements.
volatile uint16_t v_loop_Initialize(volatile struct NPNZ16b_s *controller)
Initializes controller coefficient arrays and normalization factors.
Data structure packing A- and B- coefficient arrays in a linear memory space for optimized DSP code e...
void v_loop_Reset(volatile struct NPNZ16b_s *controller)
Prototype of the Assembly routine '_v_loop_Reset' clearing the NPNZ16b controller output and error hi...
volatile int16_t PTermScaler
Q15 P-Term Coefficient Bit-Shift Scaler (R/W)
volatile int16_t v_loop_pterm_factor
Q15 fractional of the P-Term Coefficient for Plant Measurements.
volatile int16_t normPreShift
Normalization of ADC-resolution to Q15 (R/W)
volatile uint16_t BCoefficientsArraySize
Size of the B coefficients array in X-space.
Data structure packing A- and B- coefficient arrays in a linear memory space for optimized DSP code e...
volatile int16_t normPostShiftA
Normalization of A-term control output to Q15 (R/W)
volatile fractional * ptrControlHistory
Pointer to n delay-line samples located in Y-space with first sample being the most recent.
volatile uint16_t ACoefficientsArraySize
Size of the A coefficients array in X-space.
volatile int16_t normPostScaler
Control output normalization factor (Q15) (R/W)
volatile uint16_t ErrorHistoryArraySize
Size of the error history array in Y-space.
volatile int16_t normPostShiftB
Normalization of B-term control output to Q15 (R/W)
volatile int16_t PTermFactor
Q15 P-Term Coefficient Factor (R/W)
volatile int32_t * ptrBCoefficients
Pointer to B coefficients located in X-space.