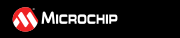 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
13 #include "dev_buck_typedef.h"
14 #include "dev_buck_opstates.h"
15 #include "dev_buck_pconfig.h"
32 volatile uint16_t retval = 1;
33 volatile uint16_t _i=0;
60 buckInstance->
state_id.
value = (uint32_t)BUCK_OPSTATE_INITIALIZE;
84 volatile uint16_t retval=1;
90 if(buckInstance == NULL)
118 buckInstance->
state_id.
value = (uint32_t)BUCK_OPSTATE_INITIALIZE;
125 return((
bool)(retval>0));
135 buckInstance->
state_id.
value = (uint32_t)BUCK_OPSTATE_INITIALIZE;
147 case BUCK_OPSRET_ERROR:
149 buckInstance->
state_id.
value = (uint32_t)BUCK_OPSTATE_INITIALIZE;
154 case BUCK_OPSRET_COMPLETE:
167 case BUCK_OPSRET_REPEAT:
177 buckInstance->
state_id.
value = (uint32_t)BUCK_OPSTATE_INITIALIZE;
199 volatile uint16_t retval=1;
200 volatile uint16_t _i=0;
222 buckInstance->
state_id.
value = (uint32_t)BUCK_OPSTATE_INITIALIZE;
242 volatile uint16_t retval=1;
243 volatile uint16_t _i=0;
256 buckInstance->
state_id.
value = (uint32_t)BUCK_OPSTATE_INITIALIZE;
275 volatile uint16_t retval=1;
296 volatile uint16_t retval=1;
volatile uint16_t BuckStateList_size
Buck converter state machine function pointer array size
volatile uint16_t(* BuckConverterStateMachine[])(volatile struct BUCK_CONVERTER_s *buckInstance)
Buck converter state machine function pointer array.
volatile uint16_t buckADC_ModuleInitialize(void)
This fucntion initializes the buck by resetting all its registers to default.
volatile struct BUCK_FEEDBACK_SETTINGS_s feedback
BUCK converter feedback settings.
enum BUCK_CONTROL_MODE_e control_mode
Fundamental control mode.
volatile struct BUCK_LOOP_SETTINGS_s i_loop[BUCK_MPHASE_COUNT]
BUCK Current control loop objects.
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
volatile bool fault_active
Bit #5: Flag bit indicating system is in enforced shut down mode (usually due to a fault condition)
volatile uint16_t polarity
Output polarity, where 0=ACTIVE HIGH, 1=ACTIVE_LOW.
volatile uint16_t drv_BuckConverter_Resume(volatile struct BUCK_CONVERTER_s *buckInstance)
This function resume the operation of the buck converter.
volatile uint16_t drv_BuckConverter_Start(volatile struct BUCK_CONVERTER_s *buckInstance)
This function starts the buck converter.
volatile bool suspend
Bit #6: Control bit to put the converter in suspend mode (turned off while ENABLE bit is still on)
volatile struct NPNZ_STATUS_s status
Control Loop Status and Control flags.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vout
ADC input sampling output voltage.
volatile uint16_t drv_BuckConverter_Initialize(volatile struct BUCK_CONVERTER_s *buckInstance)
This function initializes all peripheral modules and their instances used by the power controller.
volatile uint16_t buckADC_ChannelInitialize(volatile struct BUCK_ADC_INPUT_SETTINGS_s *adcInstance)
This function initializes the settings for the ADC channel.
volatile uint16_t drv_BuckConverter_Stop(volatile struct BUCK_CONVERTER_s *buckInstance)
This function stop the buck converter opration.
volatile uint16_t buckPWM_Start(volatile struct BUCK_CONVERTER_s *buckInstance)
This function enables the buck PWM operation.
volatile bool enabled
Specifies, if this IO is used or not.
volatile bool enabled
Bit 15: enables/disables control loop execution.
volatile bool ready
Bit #0: status bit, indicating buck converter is initialized and ready to run.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vin
ADC input sampling input voltage.
volatile struct BUCK_GPIO_INSTANCE_s EnableInput
External ENABLE input.
volatile bool buckGPIO_GetPinState(volatile struct BUCK_GPIO_INSTANCE_s *buckGPIOInstance)
This function gets the state of the selected pin.
volatile uint16_t buckPWM_Stop(volatile struct BUCK_CONVERTER_s *buckInstance)
This function stops the buck PWM output.
void(* ctrl_Reset)(volatile struct NPNZ16b_s *)
Function pointer to RESET routine.
volatile struct BUCK_GPIO_SETTINGS_s gpio
BUCK converter additional GPIO specification.
volatile uint16_t buckGPIO_Initialize(volatile struct BUCK_CONVERTER_s *buckInstance)
This function initializes the buck input pins.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_isns[BUCK_MPHASE_COUNT]
ADC input sampling phase current.
volatile struct BUCK_CONVERTER_SETTINGS_s set_values
Control field for global access to references.
volatile bool pwm_active
Bit #2: indicating that PWM has been started and ADC triggers are generated.
volatile uint16_t buckPWM_ModuleInitialize(volatile struct BUCK_CONVERTER_s *buckInstance)
Initializes the buck PWM module by resetting its registers to default.
volatile uint16_t buckADC_Start(void)
This function enables the ADC module and starts the ADC cores analog inputs for the required input si...
volatile struct NPNZ16b_s * controller
pointer to control loop object data structure
BUCK control & monitoring data structure.
struct BUCK_STATE_ID_s::@3::@4 bits
volatile struct BUCK_STATE_ID_s state_id
BUCK state machine operating state ID.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_temp
ADC input sampling temperature.
volatile struct BUCK_LOOP_SETTINGS_s v_loop
BUCK voltage control loop object.
volatile uint16_t drv_BuckConverter_Execute(volatile struct BUCK_CONVERTER_s *buckInstance)
This function is the main buck converter state machine executing the most recent state.
volatile uint16_t no_of_phases
number of converter phases
volatile uint16_t buckPWM_ChannelInitialize(volatile struct BUCK_CONVERTER_s *buckInstance)
This function initializes the output pins for the PWM output and the default buck PWM settings.
volatile uint16_t drv_BuckConverter_Suspend(volatile struct BUCK_CONVERTER_s *buckInstance)
This function suspends the operation of the buck converter.
volatile bool enabled
Bit #15: Control bit enabling/disabling the charger port.