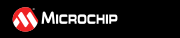 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
10 #ifndef __SPECIAL_FUNCTION_LAYER_LIB_NPNZ16B_H__
11 #define __SPECIAL_FUNCTION_LAYER_LIB_NPNZ16B_H__
38 #ifndef __PSDCLD_VERSION
39 #define __PSDCLD_VERSION 912
55 enum NPNZ_STATUS_FLAGS_e
57 NPNZ_STATUS_CLEAR = 0b0000000000000000,
58 NPNZ_STATUS_SATUATION_MSK = 0b0000000000000011,
59 NPNZ_STATUS_LSAT_ACTIVE = 0b0000000000000001,
60 NPNZ_STATUS_LSAT_CLEAR = 0b0000000000000000,
61 NPNZ_STATUS_USAT_ACTIVE = 0b0000000000000010,
62 NPNZ_STATUS_USAT_CLEAR = 0b0000000000000000,
63 NPNZ_STATUS_AGC_DISABLE = 0b0000000000000000,
64 NPNZ_STATUS_AGC_ENABLED = 0b0000100000000000,
65 NPNZ_STATUS_TARGET_DEFAULT = 0b0000000000000000,
66 NPNZ_STATUS_TARGET_SWAPED = 0b0001000000000000,
67 NPNZ_STATUS_SOURCE_DEFAULT = 0b0000000000000000,
68 NPNZ_STATUS_SOURCE_SWAPED = 0b0010000000000000,
69 NPNZ_STATUS_INV_INPUT_OFF = 0b0000000000000000,
70 NPNZ_STATUS_INV_INPUT_ON = 0b0100000000000000,
71 NPNZ_STATUS_ENABLE_OFF = 0b0000000000000000,
72 NPNZ_STATUS_ENABLE_ON = 0b1000000000000000
74 typedef enum NPNZ_STATUS_FLAGS_e NPNZ_STATUS_FLAGS_t;
80 enum NPNZ_STATUS_SATURATION_e{
84 typedef enum NPNZ_STATUS_SATURATION_e NPNZ_STATUS_SATURATION_t;
85 extern volatile enum NPNZ_STATUS_SATURATION_e npnzEnumControlStatusSaturation;
92 enum NPNZ_STATUS_AGC_ENABLE_e{
93 NPNZ_AGC_DISABLED = 0b0,
94 NPNZ_AGC_ENABLED = 0b1
96 typedef enum NPNZ_STATUS_AGC_ENABLE_e NPNZ_STATUS_AGC_ENABLE_t;
97 extern volatile enum NPNZ_STATUS_AGC_ENABLE_e npnzEnumControlAgcEnable;
103 enum NPNZ_STATUS_SOURCE_SWAP_e{
104 NPNZ_SOURCE_DEFAULT = 0b0,
105 NPNZ_SOURCE_SWAPED = 0b1
107 typedef enum NPNZ_STATUS_SOURCE_SWAP_e NPNZ_STATUS_SOURCE_SWAP_t;
108 extern volatile enum NPNZ_STATUS_SOURCE_SWAP_e npnzEnumControlSourceSwap;
114 enum NPNZ_STATUS_TARGET_SWAP_e{
115 NPNZ_TARGET_DEFAULT = 0b0,
116 NPNZ_TARGET_SWAPED = 0b1
118 typedef enum NPNZ_STATUS_TARGET_SWAP_e NPNZ_STATUS_TARGET_SWAP_t;
119 extern volatile enum NPNZ_STATUS_TARGET_SWAP_e npnzEnumControlTargetSwap;
125 enum NPNZ_STATUS_INPUT_INV_e{
126 NPNZ_INPUT_DEFAULT = 0b0,
127 NPNZ_INPUT_INVERTED = 0b1
129 typedef enum NPNZ_STATUS_INPUT_INV_e NPNZ_STATUS_INPUT_INV_t;
130 extern volatile enum NPNZ_STATUS_INPUT_INV_e npnzEnumControlInputInversion;
136 enum NPNZ_STATUS_ENABLE_e{
140 typedef enum NPNZ_STATUS_ENABLE_e NPNZ_STATUS_ENABLE_t;
141 extern volatile enum NPNZ_STATUS_ENABLE_e npnzEnumControlEnable;
203 } __attribute__((packed))bits;
206 } __attribute__((packed));
233 } __attribute__((packed));
267 } __attribute__((packed));
307 } __attribute__((packed));
334 } __attribute__((packed));
361 } __attribute__((packed));
388 } __attribute__((packed));
430 } __attribute__((packed));
458 } __attribute__((packed));
485 } __attribute__((packed));
514 } __attribute__((packed)) ;
519 #endif // end of __SPECIAL_FUNCTION_LAYER_LIB_NPNZ16B_H__ header file section
volatile uint16_t ExtHookPreAntiWindupFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
volatile uint16_t * ptrADCTriggerARegister
Pointer to ADC trigger #1 register (e.g. TRIG1)
volatile uint16_t ptrExtHookStopFunction
Pointer to Function which is called at the end of the control loop but will be bypassed when the cont...
volatile int16_t AltMaxOutput
Alternate maximum output value used for clamping (R/W)
volatile uint16_t ADCTriggerBOffset
ADC trigger #2 offset to compensate propagation delays.
volatile bool swap_source
Bit 13: when set, AltSource is used as data input to controller.
NPNZ16b controller object status and control word.
volatile uint16_t ExtHookSourceFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
volatile bool invert_input
Bit 14: when set, most recent error input value to controller is inverted.
volatile uint16_t AgcScaler
Bit-shift scaler of Adaptive Gain Modulation factor.
volatile uint16_t ExtHookTargetFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
Data Input/Output Port declaration of memory addresses, signal offsets and normalization settings.
volatile uint16_t * ptrDProvControlInputCompensated
Pointer to external data buffer of most recent, compensated control input.
volatile uint16_t usrParam7
generic 16-bit wide, user-defined parameter #8 for advanced control options
enum NPNZ_STATUS_INPUT_INV_e flagCtrlInputInversion
List of State Machine Operating State Return Values.
enum NPNZ_STATUS_FLAGS_e StatusWordFlags
List of all status and control flags of the Buck Converter status word.
Consolidated list of status bit value enumerations.
volatile int16_t Offset
Value/signal offset of this port.
Global NPNZ controller data object.
volatile int32_t * ptrACoefficients
Pointer to A coefficients located in X-space.
volatile bool lower_saturation_event
Bit 0: control loop is clamped at minimum output level.
volatile struct NPNZ_DATA_PROVIDERS_s DataProviders
Automated data sources pushing recent data points to user-defined variables.
Adaptive Gain Control Modulation Parameters.
volatile uint16_t ControlHistoryArraySize
Size of the control history array in Y-space.
enum NPNZ_STATUS_AGC_ENABLE_e flagAgcControl
List of all status and control flags of the Buck Converter status word.
volatile uint16_t usrParam4
generic 16-bit wide, user-defined parameter #5 for advanced control options
Automated ADC Trigger handling.
volatile uint16_t value
Controller status full register access.
volatile struct NPNZ_PORT_s Target
Primary data output port declaration.
volatile struct NPNZ_ADC_TRGCTRL_s ADCTriggerControl
Automatic ADC trigger placement options for ADC Trigger A and B.
volatile uint16_t usrParam1
generic 16-bit wide, user-defined parameter #2 for advanced control options
volatile uint16_t ptrExtHookEndFunction
Pointer to Function which is called at the end of the control loop and will also be called when the c...
volatile struct NPNZ_PORT_s AltTarget
Secondary data output port declaration.
volatile uint16_t ADCTriggerAOffset
ADC trigger #1 offset to compensate propagation delays.
volatile fractional * ptrErrorHistory
Pointer to n+1 delay-line samples located in Y-space with first sample being the most recent.
volatile struct NPNZ_FILTER_PARAMS_s Filter
Filter parameters such as pointer to history and coefficient arrays and number scaling.
volatile struct NPNZ_STATUS_s status
Control Loop Status and Control flags.
volatile int16_t MaxOutput
Maximum output value used for clamping (R/W)
Filter Coefficient Arrays, Number Format Handling and Input/Output History Parameters.
volatile uint16_t ptrExtHookPreAntiWindupFunction
Pointer to Function which will be called after the compensation filter computation is complete and be...
volatile uint16_t * ptrDProvControlInput
Pointer to external data buffer of most recent, raw control input.
volatile uint16_t * ptrDProvControlOutput
Pointer to external data buffer of most recent control output.
volatile int16_t MinOutput
Minimum output value used for clamping (R/W)
volatile bool upper_saturation_event
Bit 1: control loop is clamped at maximum output level.
volatile bool enabled
Bit 15: enables/disables control loop execution.
volatile uint16_t ptrAgcObserverFunction
Function Pointer to Observer function updating the AGC modulation factor.
volatile struct NPNZ_EXTENSION_HOOKS_s ExtensionHooks
User extension function triggers using function pointers with parameters.
volatile uint16_t ptrExtHookStartFunction
Pointer to Function which will be called at the beginning of the control loop.
volatile int16_t NormScaler
Bit-shift scaler of the Q15 normalization factor.
volatile uint16_t ptrExtHookTargetFunction
Pointer to Function which will be called before the most recent control output is written to target.
volatile fractional NormFactor
Q15 normalization factor.
volatile int16_t PTermScaler
Q15 P-Term Coefficient Bit-Shift Scaler (R/W)
User Extension Function Call Parameters.
volatile struct NPNZ_PORT_s AltSource
Secondary data input port declaration.
volatile int16_t normPreShift
Normalization of ADC-resolution to Q15 (R/W)
volatile uint16_t BCoefficientsArraySize
Size of the B coefficients array in X-space.
volatile bool swap_target
Bit 12: when set, AltTarget is used as data output of controller.
volatile uint16_t ExtHookStopFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
volatile uint16_t usrParam6
generic 16-bit wide, user-defined parameter #7 for advanced control options
volatile uint16_t ExtHookStartFunctionParam
Parameter of function called (can be a variable or pointer to a data structure)
volatile uint16_t usrParam0
generic 16-bit wide, user-defined parameter #1 for advanced control options
volatile int16_t normPostShiftA
Normalization of A-term control output to Q15 (R/W)
enum NPNZ_STATUS_TARGET_SWAP_e flagTargetSwap
List of State Machine Sub-State IDs.
volatile unsigned
Bit 2: reserved.
volatile bool agc_enabled
Bit 11: when set, Adaptive Gain Control Modulation is enabled.
User Data Space for Advanced Control Functions.
volatile uint16_t ptrExtHookSourceFunction
Pointer to Function which will be called after the source has been read and compensated.
Data Provider Target Memory Addresses.
enum NPNZ_STATUS_SOURCE_SWAP_e flagSourceSwap
List of State Machine Operating State IDs.
enum NPNZ_STATUS_SATURATION_e flagSaturation
List of all status and control flags of the Buck Converter status word.
enum NPNZ_STATUS_ENABLE_e flagControlEnable
List of Supported Control Modes.
volatile uint16_t usrParam2
generic 16-bit wide, user-defined parameter #3 for advanced control options
volatile fractional * ptrControlHistory
Pointer to n delay-line samples located in Y-space with first sample being the most recent.
volatile struct NPNZ_PORT_s Source
Primary data input port declaration.
volatile struct NPNZ_LIMITS_s Limits
Input and output clamping values.
volatile uint16_t ExtHookEndFunctionParam
Parameter of function called (can be a variable or a pointer to a data structure)
System Anti-Windup (Output Clamping) Thresholds.
volatile uint16_t ACoefficientsArraySize
Size of the A coefficients array in X-space.
volatile int16_t normPostScaler
Control output normalization factor (Q15) (R/W)
volatile uint16_t * ptrDProvControlError
Pointer to external data buffer of most recent control error.
volatile fractional AgcFactor
Q15 value of Adaptive Gain Modulation factor.
volatile uint16_t * ptrADCTriggerBRegister
Pointer to ADC trigger #2 register (e.g. TRIG2)
volatile uint16_t ErrorHistoryArraySize
Size of the error history array in Y-space.
volatile int16_t normPostShiftB
Normalization of B-term control output to Q15 (R/W)
volatile int16_t PTermFactor
Q15 P-Term Coefficient Factor (R/W)
volatile int32_t * ptrBCoefficients
Pointer to B coefficients located in X-space.
volatile struct NPNZ_PORTS_s Ports
Controller input and output ports.
volatile struct NPNZ_GAIN_CONTROL_s GainControl
Parameter section for advanced control options.
volatile uint16_t usrParam3
generic 16-bit wide, user-defined parameter #4 for advanced control options
volatile fractional AgcMedian
Q15 value of Adaptive Gain Modulation nominal operating point.
volatile struct NPNZ_USER_DATA_BUFFER_s Advanced
Parameter section for advanced user control options.
volatile int16_t AltMinOutput
Alternate minimum output value used for clamping (R/W)
volatile uint16_t * ptrAddress
Pointer to register or variable where the value is read from (e.g. ADCBUFx) or written to (e....
Filter Coefficient Arrays, Number Format Handling and Input/Output History Parameters.
volatile uint16_t usrParam5
generic 16-bit wide, user-defined parameter #6 for advanced control options
volatile uint16_t * ptrControlReference
Pointer to global variable of input register holding the controller reference value (e....