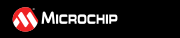 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
10 #include "drivers/v_loop.h"
11 #include "devices/dev_buck_typedef.h"
12 #include "devices/dev_buck_converter.h"
13 #include "config/hal.h"
15 #include "fault_handler/app_fault_monitor.h"
38 #define ISNS_AVG_BITMASK (uint16_t)0x0007
39 volatile uint16_t _isns_sample_count = 0;
40 volatile uint16_t isns_samples;
65 volatile uint16_t retval=1;
74 if(!(++_isns_sample_count & ISNS_AVG_BITMASK))
76 isns_samples = (isns_samples >> 3);
78 if((int16_t)isns_samples < 0) isns_samples = 0;
95 #if (PLANT_MEASUREMENT == false)
135 volatile uint16_t retval=1;
166 volatile uint16_t retval=1;
194 volatile uint16_t retval=1;
214 volatile uint16_t retval=1;
235 volatile uint16_t retval=0;
volatile uint16_t appPowerSupply_Resume(void)
This function resumes the power supply operation.
volatile struct FAULT_OBJECT_s fltobj_BuckRegErr
Regulation Error Fault Object.
#define _BUCK_VLOOP_ISR_IP
Interupt vector priority register.
#define _BUCK_VLOOP_ISR_IE
Interupt vector enable bit register bit.
volatile uint16_t v_in
BUCK input voltage.
volatile struct BUCK_LOOP_SETTINGS_s i_loop[BUCK_MPHASE_COUNT]
BUCK Current control loop objects.
volatile struct FLT_OBJECT_STATUS_s Status
Status word of this fault object.
volatile uint16_t appPowerSupply_ConverterObjectInitialize(void)
This function initializes the buck converter device driver instance.
#define BUCK_VIN_OFFSET
Input voltage feedback offset.
volatile uint16_t appPowerSupply_Stop(void)
This function calls the buck converter device driver function stopping the power supply.
volatile uint16_t appPowerSupply_ControllerInitialize(void)
This function initializes the control system feedback loop objects.
volatile uint16_t i_sns[BUCK_MPHASE_COUNT]
BUCK output current.
volatile uint16_t drv_BuckConverter_Resume(volatile struct BUCK_CONVERTER_s *buckInstance)
This function resume the operation of the buck converter.
volatile uint16_t drv_BuckConverter_Start(volatile struct BUCK_CONVERTER_s *buckInstance)
This function starts the buck converter.
volatile struct NPNZ_STATUS_s status
Control Loop Status and Control flags.
#define BUCK_VOUT_ISR_PRIORITY
Voltage loop interrupt vector priority (valid settings between 0...6 with 6 being the highest priorit...
#define BUCK_ISNS_ADCBUF
ADC input buffer of this ADC channel.
volatile uint16_t appPowerSupply_Start(void)
This function calls the buck converter device driver function starting the power supply.
volatile uint16_t drv_BuckConverter_Stop(volatile struct BUCK_CONVERTER_s *buckInstance)
This function stop the buck converter opration.
volatile struct FLT_COMPARE_OBJECT_s ReferenceObject
Reference object the source should be compared with.
volatile bool enabled
Bit 15: enables/disables control loop execution.
volatile uint16_t appPowerSupply_PeripheralsInitialize(void)
This function is used to load peripheral configuration templates from the power controller device dri...
#define _BUCK_VLOOP_ISR_IF
Interupt vector flag bit register bit.
volatile uint16_t appPowerSupply_Suspend(void)
This function stops the power supply operation.
volatile bool Enabled
Bit 15: Control bit enabling/disabling monitoring of the fault object.
#define BUCK_VIN_ADCBUF
ADC input buffer of this ADC channel.
volatile struct BUCK_CONVERTER_DATA_s data
BUCK runtime data.
volatile uint16_t * ptrObject
Pointer to register or variable which should be monitored.
volatile uint16_t i_out
BUCK common output current.
volatile uint16_t appPowerSupply_Execute(void)
This is the top-level function call triggering the most recent state machine of all associated power ...
volatile struct NPNZ16b_s * controller
pointer to control loop object data structure
volatile uint16_t temp
BUCK board temperature.
volatile struct FAULT_OBJECT_s fltobj_BuckOCP
Over Current Protection Fault Object.
BUCK control & monitoring data structure.
struct BUCK_STATE_ID_s::@3::@4 bits
volatile struct BUCK_STATE_ID_s state_id
BUCK state machine operating state ID.
volatile struct BUCK_LOOP_SETTINGS_s v_loop
BUCK voltage control loop object.
volatile uint16_t appPowerSupply_Initialize(void)
Calls the application layer power controller initialization.
#define TEMP_ADCBUF
GPIO analog function mode enable bit.
volatile uint16_t drv_BuckConverter_Execute(volatile struct BUCK_CONVERTER_s *buckInstance)
This function is the main buck converter state machine executing the most recent state.
volatile struct NPNZ_PORTS_s Ports
Controller input and output ports.
volatile struct BUCK_CONVERTER_s buck
Global data object for a BUCK CONVERTER.
volatile uint16_t drv_BuckConverter_Suspend(volatile struct BUCK_CONVERTER_s *buckInstance)
This function suspends the operation of the buck converter.
volatile uint16_t feedback_offset
Feedback offset value for calibration or bi-direction feedback signals.
volatile uint16_t * ptrControlReference
Pointer to global variable of input register holding the controller reference value (e....