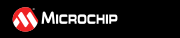 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
13 #include "dev_buck_pconfig.h"
14 #include "dev_buck_typedef.h"
52 volatile uint16_t retval=0;
64 retval = BUCK_OPSRET_COMPLETE;
69 retval = BUCK_OPSRET_REPEAT;
97 volatile uint16_t _i=0;
98 volatile uint32_t _vout=0;
99 volatile uint32_t _vin=0;
100 volatile uint32_t _start_dc=0;
130 _vout = __builtin_muluu(
135 _vin = __builtin_muluu(
141 if (_vout > _vin) _vout = _vin;
148 _start_dc = __builtin_muluu((_vout), buckInstance->
sw_node[0].
period);
149 _start_dc = __builtin_divud(_start_dc, (uint16_t)_vin);
165 if(_start_dc < buckInstance->
v_loop.minimum)
183 if(_start_dc < buckInstance->
i_loop[_i].minimum)
207 return(BUCK_OPSRET_ERROR);
210 return(BUCK_OPSRET_COMPLETE);
232 volatile uint16_t retval=1;
233 volatile uint16_t _i=0;
263 return(BUCK_OPSRET_ERROR);
280 retval = BUCK_OPSRET_COMPLETE;
286 retval = BUCK_OPSRET_REPEAT;
315 volatile uint16_t retval=0;
332 retval = BUCK_OPSRET_COMPLETE;
337 retval = BUCK_OPSRET_REPEAT;
343 retval = BUCK_OPSRET_COMPLETE;
365 volatile uint16_t retval=0;
381 return(BUCK_OPSTATE_ERROR);
384 retval = BUCK_OPSRET_COMPLETE;
389 retval = BUCK_OPSRET_REPEAT;
volatile uint16_t period
Soft-Start Period (POD, RAMP PERIOD, PGD, etc.)
volatile struct NPNZ16b_s v_loop
External reference to user-defined NPNZ16b controller data object 'v_loop'.
volatile uint16_t reference
Control loop reference variable.
volatile struct BUCK_CONVERTER_STARTUP_s startup
BUCK startup timing settings.
volatile uint16_t counter
Soft-Start Execution Counter (read only)
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s v_ramp
volatile struct BUCK_FEEDBACK_SETTINGS_s feedback
BUCK converter feedback settings.
volatile int16_t factor
Fractional scaling factor (range -1 ... 0.99969)
enum BUCK_CONTROL_MODE_e control_mode
Fundamental control mode.
volatile uint16_t v_in
BUCK input voltage.
volatile struct BUCK_LOOP_SETTINGS_s i_loop[BUCK_MPHASE_COUNT]
BUCK Current control loop objects.
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
volatile uint16_t duty_ratio_min
Absolute duty cycle minimum during normal operation.
volatile bool busy
Bit #7: Flag bit indicating that the state machine is executing a process (e.g. startup-ramp)
volatile struct NPNZ_PORT_s Target
Primary data output port declaration.
volatile uint16_t SubState_PowerGoodDelay(volatile struct BUCK_CONVERTER_s *buckInstance)
In this function, a counter is incremented until the power good delay has expired.
volatile uint16_t SubState_PowerOnDelay(volatile struct BUCK_CONVERTER_s *buckInstance)
This function delays the startup until the Power-on Delay has expired.
volatile uint16_t reference
Internal dummy reference used to increment/decrement controller reference.
volatile struct NPNZ_PORT_s AltTarget
Secondary data output port declaration.
volatile uint16_t minimum
output clamping value (minimum)
volatile struct NPNZ_STATUS_s status
Control Loop Status and Control flags.
volatile int16_t MaxOutput
Maximum output value used for clamping (R/W)
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vout
ADC input sampling output voltage.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s power_on_delay
volatile bool enabled
Specifies, if this IO is used or not.
volatile bool enabled
Bit 15: enables/disables control loop execution.
volatile uint16_t(* BuckConverterRampUpSubStateMachine[])(volatile struct BUCK_CONVERTER_s *buckInstance)
Buck converter state machine function pointer array.
volatile struct BUCK_ADC_INPUT_SETTINGS_s ad_vin
ADC input sampling input voltage.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s i_ramp
volatile struct BUCK_SWITCH_NODE_SETTINGS_s sw_node[BUCK_MPHASE_COUNT]
BUCK converter switch node settings.
volatile struct BUCK_CONVERTER_DATA_s data
BUCK runtime data.
volatile int16_t offset
Signal offset as signed integer to be subtracted from ADC input.
volatile struct BUCK_GPIO_SETTINGS_s gpio
BUCK converter additional GPIO specification.
volatile uint16_t maximum
output clamping value (maximum)
void(* ctrl_Precharge)(volatile struct NPNZ16b_s *, volatile fractional, volatile fractional)
Function pointer to PRECHARGE routine.
volatile uint16_t SubState_PrepareVRampUp(volatile struct BUCK_CONVERTER_s *buckInstance)
This function calculate and pre-charge PWM outputs with ideal duty cycle.
volatile uint16_t v_ref
User reference setting used to control the power converter controller.
volatile int16_t scaler
Feedback bit-shift scaler used for number normalization.
volatile uint16_t buckPWM_Resume(volatile struct BUCK_CONVERTER_s *buckInstance)
This function resumes the buck PWM operation.
volatile uint16_t ref_inc_step
Size/value of one reference increment/decrement or this period.
volatile struct BUCK_CONVERTER_SETTINGS_s set_values
Control field for global access to references.
volatile struct BUCK_GPIO_INSTANCE_s PowerGood
Power Good Output.
volatile uint16_t BuckRampUpSubStateList_size
Buck converter sub-state machine function pointer array size.
volatile uint16_t i_ref
User reference setting used to control the power converter controller.
volatile struct NPNZ16b_s * controller
pointer to control loop object data structure
volatile struct NPNZ_LIMITS_s Limits
Input and output clamping values.
BUCK control & monitoring data structure.
volatile struct BUCK_STARTUP_PERIOD_HANDLER_s power_good_delay
volatile uint16_t SubState_IRampUp(volatile struct BUCK_CONVERTER_s *buckInstance)
This function is for the average current mode control where the output current is ramped up to nomina...
volatile struct BUCK_LOOP_SETTINGS_s v_loop
BUCK voltage control loop object.
volatile uint16_t buckGPIO_Set(volatile struct BUCK_GPIO_INSTANCE_s *buckGPIOInstance)
This function sets the selected general purpose input/ouput pins.
volatile uint16_t SubState_VRampUp(volatile struct BUCK_CONVERTER_s *buckInstance)
This function ramps up the output voltage to its nominal regulation point.
volatile struct NPNZ_PORTS_s Ports
Controller input and output ports.
volatile struct BUCK_ADC_INPUT_SCALING_s scaling
normalization scaling settings
volatile uint16_t no_of_phases
number of converter phases
volatile uint16_t period
Switching period.
volatile uint16_t * ptrAddress
Pointer to register or variable where the value is read from (e.g. ADCBUFx) or written to (e....
volatile uint16_t v_out
BUCK output voltage.
volatile uint16_t * ptrControlReference
Pointer to global variable of input register holding the controller reference value (e....