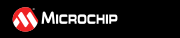 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
8 #if (__XC16_VERSION__ > 1500)
9 #pragma message ("Warning: Library file '" __FILE__ "' has not been tested with the recently selected compiler version")
16 #include "dev_buck_pconfig.h"
17 #include "dev_buck_templates.h"
56 volatile uint16_t retval=1;
60 volatile bool _pmdlock = PMDCONbits.PMDLOCK;
61 PMDCONbits.PMDLOCK = 0;
63 PMDCONbits.PMDLOCK = _pmdlock;
112 volatile uint16_t retval=1;
113 volatile uint16_t _i=0;
117 volatile uint16_t pwm_Instance;
118 volatile uint16_t gpio_Instance;
214 volatile uint16_t retval=1;
215 volatile uint16_t _i=0;
216 volatile uint16_t timeout=0;
217 volatile uint16_t pwm_Instance=0;
218 volatile uint16_t sync_sw_mask=0;
238 while((!PCLKCONbits.HRRDY) && (timeout++ < 5000));
239 if ((timeout >= 5000) || (PCLKCONbits.HRERR))
260 retval &= (
volatile uint16_t)(pg->
PGxCONL.
bits.ON);
283 volatile uint16_t retval=1;
284 volatile uint16_t _i=0;
285 volatile uint16_t pwm_Instance=0;
303 retval &= (
volatile uint16_t)((
volatile bool)(pg->
PGxCONL.
bits.ON == 0));
329 volatile uint16_t retval=1;
330 volatile uint16_t _i=0;
331 volatile uint16_t pwm_Instance;
371 volatile uint16_t retval=1;
372 volatile uint16_t _i=0;
373 volatile bool sync_mode=
false;
374 volatile uint16_t pwm_Instance=0;
375 volatile uint16_t sync_sw_mask=0;
408 retval &= (uint16_t)((
bool)(!(pg->
PGxIOCONL.
value & sync_sw_mask)));
431 volatile uint16_t retval=1;
434 volatile bool _pmdlock = PMDCONbits.PMDLOCK;
435 PMDCONbits.PMDLOCK = 0;
437 PMDCONbits.PMDLOCK = _pmdlock;
440 ADCON1Lbits.ADON = 0;
441 ADCON1Lbits.ADSIDL = 0;
444 ADCON1Hbits.SHRRES = 0b11;
445 ADCON1Hbits.FORM = 0;
448 ADCON2Lbits.REFCIE = 0;;
449 ADCON2Lbits.REFERCIE = 0;
450 ADCON2Lbits.EIEN = 1;
451 ADCON2Lbits.SHREISEL = 0b111;
452 ADCON2Lbits.SHRADCS = 0b0000001;
455 ADCON2Hbits.SHRSAMC = 8;
456 ADCON2Hbits.REFERR = 0;
457 ADCON2Hbits.REFRDY = 0;
460 ADCON3Lbits.REFSEL = 0b000;
461 ADCON3Lbits.SUSPEND = 0;
462 ADCON3Lbits.SUSPCIE = 0;
463 ADCON3Lbits.SUSPRDY = 0;
464 ADCON3Lbits.SHRSAMP = 0;
465 ADCON3Lbits.CNVRTCH = 0;
466 ADCON3Lbits.SWLCTRG = 0;
467 ADCON3Lbits.SWCTRG = 0;
468 ADCON3Lbits.CNVCHSEL = 0;
471 ADCON3Hbits.CLKSEL = 0b01;
472 ADCON3Hbits.CLKDIV = 0b000000;
473 ADCON3Hbits.SHREN = 0;
474 ADCON3Hbits.C0EN = 0;
475 ADCON3Hbits.C1EN = 0;
478 ADCON4Lbits.SAMC0EN = 0;
479 ADCON4Lbits.SAMC1EN = 0;
482 ADCON4Hbits.C0CHS = 0b00;
483 ADCON4Hbits.C1CHS = 0b00;
489 ADCON5Lbits.SHRPWR = 0;
490 ADCON5Lbits.C0PWR = 0;
491 ADCON5Lbits.C1PWR = 0;
494 ADCON5Hbits.WARMTIME = 0b1111;
495 ADCON5Hbits.SHRCIE = 0;
496 ADCON5Hbits.C0CIE = 0;
497 ADCON5Hbits.C1CIE = 0;
500 ADCORE1Lbits.SAMC = 0b0000000000;
501 ADCORE0Lbits.SAMC = 0b0000000000;
504 ADCORE0Hbits.RES = 0b11;
505 ADCORE0Hbits.ADCS = 0b0000000;
506 ADCORE0Hbits.EISEL = 0b111;
508 ADCORE1Hbits.RES = 0b11;
509 ADCORE1Hbits.ADCS = 0b0000000;
510 ADCORE1Hbits.EISEL = 0b111;
530 volatile uint16_t retval=1;
531 volatile uint8_t* ptrADCRegister;
532 volatile uint8_t bit_offset;
554 bit_offset = (2 * adcInstance->
adc_input);
556 bit_offset = (2 * (adcInstance->
adc_input-8));
558 bit_offset = (2 * (adcInstance->
adc_input-16));
560 bit_offset = (2 * (adcInstance->
adc_input-24));
564 ptrADCRegister = (
volatile uint8_t *)
565 ((
volatile uint8_t *)&ADMOD0L + (
volatile uint8_t)(adcInstance->
adc_input >> 8));
567 *ptrADCRegister |= ((
unsigned int)adcInstance->
signed_result << bit_offset);
568 *ptrADCRegister |= ((
unsigned int)adcInstance->
differential_input << (bit_offset + 1));
571 ptrADCRegister = (
volatile uint8_t *)
572 ((
volatile uint8_t *)&ADTRIG0L + (
volatile uint8_t)adcInstance->
adc_input);
624 volatile uint16_t retval=1;
625 volatile uint16_t timeout=0;
626 volatile uint16_t adcore_mask_compare=0;
629 ADCON1Lbits.ADON = 1;
655 volatile uint16_t retval=1;
656 volatile uint16_t filter_mask=0;
663 filter_mask = (0x0001 << buckGPIOInstance->
pin);
666 if (buckGPIOInstance->
polarity == 0)
690 volatile uint16_t retval=1;
691 volatile uint16_t filter_mask=0;
698 filter_mask = (0x0001 << buckGPIOInstance->
pin);
701 if (buckGPIOInstance->
polarity == 0)
725 volatile bool retval=1;
732 retval = (bool)(gpio->
PORTx.
value & (0x0001 << buckGPIOInstance->
pin));
756 volatile uint16_t retval=1;
788 volatile uint16_t retval=1;
795 if (buckGPIOInstance->
polarity == 0)
801 if(buckGPIOInstance->
io_type == 1)
807 if(buckGPIOInstance->
io_type == 2)
union P33C_GPIO_INSTANCE_s::@6 CNPDx
volatile bool async_mode
Bit #9: Control bit suspending the synchronous rectifier switch PWM channel.
#define ADC_POWERUP_TIMEOUT
Digital-To-Analog Converter and High Speed Comparator Special Function Register Set.
volatile bool differential_input
input channel differential mode enable bit
volatile bool interrupt_enable
input channel interrupt enable bit
#define ADC_CORE3_MASK_INDEX
This define masks core 3 of ADC.
struct tagPG1CONLBITS bits
#define ADC_SHRCORE_MASK_INDEX
This define masks shared core of ADC.
volatile uint16_t gpio_high
GPIO port pin-number of PWMxH of the selected PWM generator.
volatile uint16_t buckADC_ModuleInitialize(void)
This fucntion initializes the buck by resetting all its registers to default.
volatile uint16_t dead_time_rising
Dead time setting at rising edge of a half-bridge drive.
union P33C_PWM_GENERATOR_s::@58 PGxIOCONH
union P33C_GPIO_INSTANCE_s::@0 ANSELx
volatile bool swap_outputs
Selecting if PWMxH (default) or PWMxL should be the leading PWM output.
union P33C_PWM_GENERATOR_s::@56 PGxSTAT
volatile uint16_t p33c_PwmGenerator_ConfigWrite(volatile uint16_t pgInstance, volatile struct P33C_PWM_GENERATOR_s pgConfig)
Disposes a given PWM generator by resetting all its registers to default.
union P33C_GPIO_INSTANCE_s::@3 LATx
volatile uint16_t buckGPIO_Clear(volatile struct BUCK_GPIO_INSTANCE_s *buckGPIOInstance)
This function clears the selected general purpose input/output pin.
volatile uint16_t p33c_PwmModule_ConfigWrite(volatile struct P33C_PWM_MODULE_s pwmConfig)
Writes a user-defined configuration to the PWM base module registers.
union P33C_PWM_GENERATOR_s::@78 PGxDTL
volatile uint16_t buckPWM_Suspend(volatile struct BUCK_CONVERTER_s *buckInstance)
This function disables the PWM generator IOs.
volatile uint16_t io_type
Input/Output definition (0=push-pull output, 1=input, 2=open-drain output)
#define P33C_PGxSTAT_UPDREQ
Control bit in PGxSTAT setting the Update Request bit.
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
volatile uint8_t adc_core
number of the ADC core connected to the selected channel
volatile uint16_t gpio_instance
GPIO instance of the selected PWM generator.
volatile uint16_t gpio_low
GPIO port pin-number of PWMxL of the selected PWM generator.
volatile uint8_t trigger_source
input channel trigger source
volatile struct P33C_PWM_MODULE_s * p33c_PwmModule_GetHandle(void)
Gets pointer to PWM module SFR set.
volatile uint16_t duty_ratio_min
Absolute duty cycle minimum during normal operation.
#define ADC_CORE0_MASK_INDEX
This define masks core 0 of ADC.
#define P33C_PGxCONL_PWM_ON
control bit in PGxCONL enabling/disabling the PWM generator
ADC input channel configuration.
volatile bool enabled
input channel enable bit
volatile struct P33C_PWM_GENERATOR_s * p33c_PwmGenerator_GetHandle(volatile uint16_t pgInstance)
Returns the PWM generator index.
union P33C_PWM_GENERATOR_s::@69 PGxLEBL
volatile bool sync_drive
Selecting if switch node is driven in synchronous or asnchronous mode.
#define P33C_PGxIOCONH_PEN_ASYNC_SWAP
#define P33C_PGxIOCONH_PEN_ASYNC
volatile uint16_t polarity
Output polarity, where 0=ACTIVE HIGH, 1=ACTIVE_LOW.
#define P33C_PGxIOCONL_OVREN_SYNC
control bits in PGxIOCONL enabling/disabling the PWM output override in synchronous mode
volatile uint8_t adc_input
number of the ADC input channel used
volatile bool level_trigger
input channel level trigger mode enable bit
volatile uint16_t pwm_instance
number of the PWM channel used
volatile struct P33C_GPIO_INSTANCE_s * p33c_GpioInstance_GetHandle(volatile uint16_t gpioInstance)
Gets pointer to GPIO Instance SFR set.
volatile uint16_t buckADC_ChannelInitialize(volatile struct BUCK_ADC_INPUT_SETTINGS_s *adcInstance)
This function initializes the settings for the ADC channel.
union P33C_GPIO_INSTANCE_s::@1 TRISx
#define ADC_CORE1_MASK_INDEX
This define masks core 1 of ADC.
volatile uint16_t buckPWM_Start(volatile struct BUCK_CONVERTER_s *buckInstance)
This function enables the buck PWM operation.
volatile bool enabled
Specifies, if this IO is used or not.
union P33C_PWM_GENERATOR_s::@79 PGxDTH
volatile uint16_t phase
Switching signal phase-shift.
volatile struct BUCK_SWITCH_NODE_SETTINGS_s sw_node[BUCK_MPHASE_COUNT]
BUCK converter switch node settings.
volatile struct BUCK_GPIO_INSTANCE_s EnableInput
External ENABLE input.
volatile bool buckGPIO_GetPinState(volatile struct BUCK_GPIO_INSTANCE_s *buckGPIOInstance)
This function gets the state of the selected pin.
#define ADC_CORE2_MASK_INDEX
This define masks core 2 of ADC.
volatile uint16_t port
GPIO port instance number (0=Port RA, 0=Port RB, 0=Port RC, etc.)
volatile uint16_t buckPWM_Stop(volatile struct BUCK_CONVERTER_s *buckInstance)
This function stops the buck PWM output.
#define P33C_PGxCONL_HRES_EN
control bit in PGxCONL enabling/disabling High Resolution Mode
volatile struct BUCK_GPIO_SETTINGS_s gpio
BUCK converter additional GPIO specification.
union P33C_PWM_GENERATOR_s::@55 PGxCONH
volatile struct P33C_PWM_GENERATOR_s buckPwmGeneratorConfig
PWM generator default configuration.
union P33C_GPIO_INSTANCE_s::@2 PORTx
union P33C_PWM_GENERATOR_s::@74 PGxPER
volatile uint16_t buckGPIO_Initialize(volatile struct BUCK_CONVERTER_s *buckInstance)
This function initializes the buck input pins.
volatile struct P33C_PWM_MODULE_s buckPwmModuleConfig
PWM module default configuration.
volatile uint16_t buckGPIO_PrivateInitialize(volatile struct BUCK_GPIO_INSTANCE_s *buckGPIOInstance)
This function sets the pin as input or output.
volatile uint16_t buckPWM_Resume(volatile struct BUCK_CONVERTER_s *buckInstance)
This function resumes the buck PWM operation.
volatile struct BUCK_CONVERTER_SETTINGS_s set_values
Control field for global access to references.
volatile uint16_t pin
GPIO port pin number.
volatile struct BUCK_GPIO_INSTANCE_s PowerGood
Power Good Output.
union P33C_PWM_GENERATOR_s::@59 PGxEVTL
volatile bool master_period_enable
Selecting MASTER or Individual period register.
volatile uint16_t leb_period
Leading-Edge Blanking period.
union P33C_PWM_GENERATOR_s::@54 PGxCONL
volatile bool early_interrupt_enable
input channel early interrupt enable bit
volatile uint16_t buckPWM_ModuleInitialize(volatile struct BUCK_CONVERTER_s *buckInstance)
Initializes the buck PWM module by resetting its registers to default.
union P33C_PWM_GENERATOR_s::@77 PGxTRIGC
#define P33C_PGxIOCONH_PEN_SYNC
control bits in PGxIOCONH enabling/disabling the PWM outputs in synchronous mode
volatile uint16_t buckADC_Start(void)
This function enables the ADC module and starts the ADC cores analog inputs for the required input si...
volatile uint16_t adcore_diff_mask
This variable is use to set the ADC core mask.
BUCK control & monitoring data structure.
volatile uint16_t dead_time_falling
Dead time setting at falling edge of a half-bridge drive.
union P33C_GPIO_INSTANCE_s::@4 ODCx
volatile uint16_t buckGPIO_Set(volatile struct BUCK_GPIO_INSTANCE_s *buckGPIOInstance)
This function sets the selected general purpose input/ouput pins.
#define P33C_PGxIOCONL_OVREN_ASYNC_SWAP
GPIO instance of the converter control GPIO.
union P33C_PWM_GENERATOR_s::@57 PGxIOCONL
volatile uint16_t no_of_phases
number of converter phases
volatile uint16_t period
Switching period.
volatile bool signed_result
input channel singed result mode enable bit
union P33C_PWM_GENERATOR_s::@72 PGxDC
#define P33C_PGxIOCONL_OVREN_ASYNC
control bits in PGxIOCONL enabling/disabling the PWM output override in asynchronous mode
volatile uint16_t buckPWM_ChannelInitialize(volatile struct BUCK_CONVERTER_s *buckInstance)
This function initializes the output pins for the PWM output and the default buck PWM settings.
volatile bool high_resolution_enable
Selecting if PWM module should use high-resolution mode.
volatile uint16_t adcore_mask
This variable is use to set the ADC core mask.
union P33C_PWM_MODULE_s::@5 vMPER