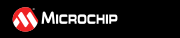 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
38 #ifndef P33C_GPIO_SFR_ABSTRACTION_H
39 #define P33C_GPIO_SFR_ABSTRACTION_H
49 #ifndef P33C_GPIO_INSTANCE_s
53 volatile struct tagLATABITS
bits;
57 volatile struct tagLATABITS
bits;
58 volatile uint16_t
value;
61 volatile struct tagLATABITS
bits;
62 volatile uint16_t
value;
65 volatile struct tagLATABITS
bits;
66 volatile uint16_t
value;
69 volatile struct tagLATABITS
bits;
70 volatile uint16_t
value;
73 volatile struct tagLATABITS
bits;
74 volatile uint16_t
value;
77 volatile struct tagLATABITS
bits;
78 volatile uint16_t
value;
81 volatile struct tagLATABITS
bits;
82 volatile uint16_t
value;
85 volatile struct tagLATABITS
bits;
86 volatile uint16_t
value;
89 volatile struct tagLATABITS
bits;
90 volatile uint16_t
value;
93 volatile struct tagLATABITS
bits;
94 volatile uint16_t
value;
97 volatile struct tagLATABITS
bits;
98 volatile uint16_t
value;
100 } __attribute__((packed));
103 #define P33C_GPIO_SFR_OFFSET ((volatile uint16_t)&ANSELB - (volatile uint16_t)&ANSELA)
112 volatile uint16_t gpio_Instance
116 volatile uint16_t gpioInstance
120 volatile uint16_t gpioInstance
124 volatile uint16_t gpioInstance,
volatile struct tagLATABITS bits
union P33C_GPIO_INSTANCE_s::@6 CNPDx
union P33C_GPIO_INSTANCE_s::@11 CNFx
union P33C_GPIO_INSTANCE_s::@0 ANSELx
union P33C_GPIO_INSTANCE_s::@3 LATx
volatile uint16_t p33c_GpioInstance_Dispose(volatile uint16_t gpioInstance)
Resets all GPIO Instance registers to their RESET default values.
union P33C_GPIO_INSTANCE_s::@9 CNSTATx
volatile uint16_t p33c_GpioInstance_ConfigWrite(volatile uint16_t gpioInstance, volatile struct P33C_GPIO_INSTANCE_s gpioConfig)
Writes a user-defined configuration to the GPIO instance registers.
volatile struct P33C_GPIO_INSTANCE_s * p33c_GpioInstance_GetHandle(volatile uint16_t gpio_Instance)
Gets pointer to GPIO Instance SFR set.
volatile struct P33C_GPIO_INSTANCE_s gpioConfigClear
Default RESET configuration of one GPIO instance SFRs.
union P33C_GPIO_INSTANCE_s::@1 TRISx
union P33C_GPIO_INSTANCE_s::@7 CNCONx
union P33C_GPIO_INSTANCE_s::@5 CNPUx
union P33C_GPIO_INSTANCE_s::@2 PORTx
union P33C_GPIO_INSTANCE_s::@10 CNEN1x
union P33C_GPIO_INSTANCE_s::@8 CNEN0x
union P33C_GPIO_INSTANCE_s::@4 ODCx
volatile struct P33C_GPIO_INSTANCE_s p33c_GpioInstance_ConfigRead(volatile uint16_t gpioInstance)
Read the current configuration from the GPIO instance registers