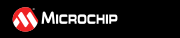 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
8 #include "lcd/app_lcd.h"
12 #include "config/apps.h"
13 #include "config/hal.h"
17 volatile struct LCD_s lcd;
47 #define LCD_STARTUP 30000
59 #define LCD_REFRESH 2000
72 #define LCD_NO_OF_SCREENS 3
90 volatile uint16_t retval = 1;
122 volatile uint16_t retval = 1;
123 volatile float vi=0.0, vo=0.0, isns=0.0, temp=0.0;
137 vi = (float)(
int)(100.0 * vi);
141 if((
double)vi < 10.000)
142 PrintLcd(0,
"VIN = %2.2f V", (
double)vi);
144 PrintLcd(0,
"VIN = %2.1f V", (
double)vi);
151 temp = (float)(
int)(100.0 * temp);
154 if((
double)temp < 10.000)
155 PrintLcd(1,
"TEMP = %2.2f C", (
double)temp);
157 PrintLcd(1,
"TEMP = %2.1f C", (
double)temp);
164 if((
double)isns < 1.000)
167 PrintLcd(1,
"ISNS = %3d mA", (
int)isns);
171 PrintLcd(1,
"ISNS = %1.2f A", (
double)isns);
176 PrintLcd(1,
"Firmware: %s", FIRMWARE_VERSION_STRING);
183 if((
double)vo < 10.000)
184 PrintLcd(1,
"VOUT = %2.2f V", (
double)vo);
186 PrintLcd(1,
"VOUT = %2.1f V", (
double)vo);
229 volatile uint16_t retval = 1;
volatile uint16_t appLCD_Initialize(void)
Initializes the LC display.
#define TEMP_FB_ZERO
Conversion macros of temperature feedback parameters.
#define BUCK_ISNS_FEEDBACK_GAIN
Current Gain in V/A.
volatile uint16_t lcd_cnt
Local counter used to trigger LCD refresh event.
volatile struct FAULT_OBJECT_s fltobj_BuckRegErr
Regulation Error Fault Object.
#define LCD_NO_OF_SCREENS
Number of screens which can be selected.
Declaration of public LC display data object.
volatile uint16_t v_in
BUCK input voltage.
volatile struct FAULT_OBJECT_s fltobj_BuckUVLO
Under Voltage Lock Out Fault Object.
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
#define PrintLcd(LINE,...)
Writes a complete line to the LC display.
volatile struct FLT_OBJECT_STATUS_s Status
Status word of this fault object.
void dev_Lcd_Initialize(void)
Initializes the LCD Device.
volatile bool fault_active
Bit #5: Flag bit indicating system is in enforced shut down mode (usually due to a fault condition)
void dev_Lcd_WriteStringXY(volatile uint8_t column_index, volatile uint8_t line_index, const char *str)
Sets the cursor position to the given x- and y-coordinates and writes the given string on the lcd scr...
volatile uint16_t refresh
volatile uint16_t appLCD_Dispose(void)
Unloads the LC display data object and resources.
volatile struct BUCK_CONVERTER_DATA_s data
BUCK runtime data.
volatile uint16_t appLCD_Execute(void)
Refreshes the LC display.
volatile uint16_t i_out
BUCK common output current.
#define LCD_REFRESH
Screen refresh delay compare value.
volatile struct FAULT_OBJECT_s fltobj_BuckOVLO
Over Voltage Lock Out Fault Object.
volatile uint16_t screens
volatile bool FaultStatus
Bit 0: Flag bit indicating if FAULT has been tripped.
volatile uint16_t temp
BUCK board temperature.
volatile struct FAULT_OBJECT_s fltobj_BuckOCP
Over Current Protection Fault Object.
#define ADC_GRANULARITY
ADC granularity in [V/tick].
volatile struct BUCK_CONVERTER_s buck
Global data object for a BUCK CONVERTER.
#define LCD_STARTUP
Startup screen delay compare value.
volatile uint16_t v_out
BUCK output voltage.