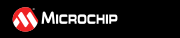 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
27 #include "common/delay.h"
28 #include "lcd/drivers/drv_lcd_interface.h"
43 #define LCD_ADDRESS_LINE_1 0x00
44 #define LCD_ADDRESS_LINE_2 0x40
46 #define LCD_DISPLAYSIZE_X 16
47 #define LCD_DISPLAYSIZE_Y 2
49 #define LCD_CLEAR 0x01
52 #define CURSOR_nSHIFT 0x00
53 #define CURSOR_SHIFT 0x01
54 #define DATA_DECREMENT 0x00
55 #define DATA_INCREMENT 0x02
56 #define LCD_ENTRY_MODE 0x04
58 #define CURSOR_OFF 0x00
59 #define CURSOR_ON 0x02
60 #define BLINK_OFF 0x00
62 #define LCD_DISPLAY_OFF 0x08
63 #define LCD_DISPLAY_ON 0x0C
65 #define FUNCTION_nIS 0x00
66 #define FUNCTION_IS 0x01
67 #define FUNCTION_1_HIGH 0x00
68 #define FUNCTION_2_HIGH 0x04
69 #define FUNCTION_1_LINE 0x00
70 #define FUNCTION_2_LINE 0x08
71 #define FUNCTION_4BITS 0x00
72 #define FUNCTION_8BITS 0x10
74 #define LCD_FUNCTION 0x20
76 #define LCD_CGRAM_ADDRESS(adr) (0x40 | (adr & 0x3F))
77 #define LCD_DDRAM_ADDRESS(adr) (0x80 | (adr & 0x7F))
82 #define FREQ_CNTRL(f) (f&0x07)
83 #define LCD_OSC_FREQ 0x10
85 #define LCD_ICON_ADDRESS(adr) (0x40 | (adr & 0x0F))
91 #define CONTRAST(c) (c&0x03)
92 #define LCD_PWR_CONTROL 0x50
94 #define FOLLOWER_GAIN(g) (g&0x07)
95 #define LCD_FOLLOWER_OFF 0x60
96 #define LCD_FOLLOWER_ON 0x68
98 #define LCD_CONTRAST(c) (0x70 | (c & 0x0F))
100 #define LCD_BUSY_FLAG_MASK 0x80
101 #define LCD_ADDRESS_MASK 0x7F
240 drv_LcdInterface_SendChar(ch);
const uint8_t line_address[]
#define LCD_DDRAM_ADDRESS(adr)
void dev_Lcd_WriteChar(const char ch)
Writes a character on the LCD screen.
#define LCD_ADDRESS_LINE_1
Newhaven NHD-C0216CZ-FSW-FBW LCD controller command set.
#define LCD_DISPLAYSIZE_Y
void drv_LcdInterface_Initialize(void)
Initializes the LCD interface driver.
void dev_Lcd_Initialize(void)
Initializes the LCD Device.
void dev_Lcd_WriteStringXY(volatile uint8_t column_index, volatile uint8_t line_index, const char *str)
Sets the cursor position to the given x- and y-coordinates and writes the given string on the lcd scr...
void drv_LcdInterface_SendCmd(uint8_t cmd)
Sends a command to the LCD controller.
#define LCD_ADDRESS_LINE_2
void dev_Lcd_GotoXY(volatile uint8_t x, volatile uint8_t y)
Sets the cursor position to the given x- and y-coordinates.
void dev_Lcd_Clear(void)
Clears the LC Display Screen.
void drv_LcdInterface_Reset(void)
Resets the LCD controller.
void dev_Lcd_WriteString(const char *str)
Writes a complete string on the LCD screen.
#define LCD_DISPLAYSIZE_X