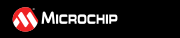 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
24 #ifndef __SPECIAL_FUNCTION_LAYER_V_LOOP_H__
25 #define __SPECIAL_FUNCTION_LAYER_V_LOOP_H__
57 } __attribute__((packed));
69 } __attribute__((packed));
155 volatile fractional ctrl_input,
156 volatile fractional ctrl_output
207 #endif // end of __SPECIAL_FUNCTION_LAYER_V_LOOP_H__
volatile struct NPNZ16b_s v_loop
External reference to user-defined NPNZ16b controller data object 'v_loop'.
void v_loop_Precharge(volatile struct NPNZ16b_s *controller, volatile fractional ctrl_input, volatile fractional ctrl_output)
Prototype of the Assembly routine '_v_loop_Precharge' loading user-defined values into the NPNZ16b co...
Global NPNZ controller data object.
volatile fractional ErrorHistory[5]
void v_loop_PTermUpdate(volatile struct NPNZ16b_s *controller)
Prototype of the alternate Assembly P-Term control loop helping to call the v_loop P-Term controller ...
volatile int16_t v_loop_pterm_scaler
Bit-shift scaler of the P-Term Coefficient for Plant Measurements.
volatile int32_t ACoefficients[4]
volatile uint16_t v_loop_Initialize(volatile struct NPNZ16b_s *controller)
Initializes controller coefficient arrays and normalization factors.
Data structure packing A- and B- coefficient arrays in a linear memory space for optimized DSP code e...
void v_loop_Reset(volatile struct NPNZ16b_s *controller)
Prototype of the Assembly routine '_v_loop_Reset' clearing the NPNZ16b controller output and error hi...
volatile int16_t v_loop_pterm_factor
Q15 fractional of the P-Term Coefficient for Plant Measurements.
Data structure packing A- and B- coefficient arrays in a linear memory space for optimized DSP code e...
void v_loop_Update(volatile struct NPNZ16b_s *controller)
Prototype of the Assembly feedback control loop routine helping to call the v_loop controller from C-...
volatile int32_t BCoefficients[5]
volatile fractional ControlHistory[4]