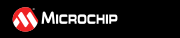 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
15 volatile bool run_main =
true;
17 #define TMR1_TIMEOUT 30000 // Timeout protection for Timer1 interrupt flag bit
18 volatile bool LOW_PRIORITY_GO =
false;
82 volatile uint16_t retval = 1;
83 volatile uint16_t timeout = 0;
107 while ((!LOW_PRIORITY_GO) && (timeout++ < TMR1_TIMEOUT));
108 LOW_PRIORITY_GO =
false;
144 volatile uint16_t retval=1;
174 volatile uint16_t retval=1;
194 void __attribute__((__interrupt__, context, no_auto_psv))
_OsTimerInterrupt(
void)
196 volatile uint16_t retval=1;
204 LOW_PRIORITY_GO =
true;
volatile uint16_t sysUserTasks_Initialize(void)
Initializes the user-defined tasks.
#define _OSTIMER_IF
interrupt flag bit
volatile uint16_t appPushButton_Execute(void)
Executes the USER push button monitor.
volatile uint16_t sysLowPriorityTasks_Execute(void)
Low priority task sequence executed after the high priority task sequence execution is complete.
volatile uint16_t sysOsTimer_Enable(volatile bool interrupt_enable, volatile uint8_t interrupt_priority)
#define DBGPIN1_Set()
Macro instruction to set a pin state to logic HIGH.
#define _OSTIMER_PRIORITY
interrupt priority (1 ... 7, default = 2)
#define _OsTimerInterrupt
Global state-machine peripheral assignments.
volatile uint16_t appLCD_Execute(void)
Refreshes the LC display.
volatile uint16_t appLED_Execute(void)
Executes the debugging LED driver.
volatile uint16_t SYSTEM_Initialize(void)
Initializes essential chip resources.
volatile uint16_t appPowerSupply_Execute(void)
This is the top-level function call triggering the most recent state machine of all associated power ...
volatile uint16_t sysUserPeriperhals_Initialize(void)
Initializes the user-defined chip resources.
volatile uint16_t appFaultMonitor_Execute(void)
Application wide fault object monitoring routine.
volatile uint16_t sysHighPriorityTasks_Execute(void)
High priority task sequence executed at a fixed repetition frequency.
#define DBGPIN1_Clear()
Macro instruction to set a pin state to logic LOW.