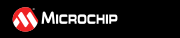 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
9 #include "config/apps.h"
10 #include "config/hal.h"
13 volatile uint16_t tgl_cnt = 0;
14 #define TGL_INTERVAL 4999 // LED toggle interval of (4999 + 1) x 100usec = 500ms
15 #define TGL_INTERVAL_ERR 999 // LED toggle interval of ( 999 + 1) x 100usec = 100ms
32 volatile uint16_t retval = 1;
35 debug_led.
period = TGL_INTERVAL;
55 volatile uint16_t retval = 1;
59 debug_led.
period = TGL_INTERVAL_ERR;
61 debug_led.
period = TGL_INTERVAL;
64 if (tgl_cnt++ > debug_led.
period) {
85 volatile uint16_t retval = 1;
volatile uint16_t appLED_Initialize(void)
Initializes the LED driving GPIO.
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
volatile bool fault_active
Bit #5: Flag bit indicating system is in enforced shut down mode (usually due to a fault condition)
volatile uint16_t appLED_Dispose(void)
Frees the resources of the debugging LED driver.
#define DBGLED_Init()
Macro instruction initializing the specified GPIO as output.
volatile uint16_t appLED_Execute(void)
Executes the debugging LED driver.
#define DBGLED_Dispose()
Macro instruction initializing the specified GPIO as input.
Debugging LED settings data object.
volatile struct BUCK_CONVERTER_s buck
Global data object for a BUCK CONVERTER.
#define DBGLED_Toggle()
Macro instruction to toggle most recent pin state.