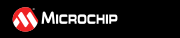 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
13 #include "xc16_pral.h"
14 #include "dev_buck_typedef.h"
15 #include "dev_buck_special_functions.h"
22 struct CS_CALIBRATION_s
26 volatile uint16_t counter;
27 volatile uint32_t buffer;
29 typedef struct CS_CALIBRATION_s CS_CALIBRATION_t;
53 #define CS_CALIB_STEPS 8
138 volatile uint16_t retval=0;
144 if(buckInstance == NULL)
180 volatile uint16_t _i=0;
181 volatile bool _complete=
true;
187 return((uint16_t)BUCK_OPSRET_COMPLETE);
194 {
return((uint16_t)BUCK_OPSRET_REPEAT); }
235 retval = (uint16_t)BUCK_OPSRET_COMPLETE;
237 retval = (uint16_t)BUCK_OPSRET_REPEAT;
volatile uint16_t(* BuckConverterSpecialFunctions[])(volatile struct BUCK_CONVERTER_s *buckInstance)
Function pointer list of all special function sub-state functions.
volatile uint16_t drv_BuckConverter_SpecialFunctionExecute(volatile struct BUCK_CONVERTER_s *buckInstance, volatile enum BUCK_SPECIAL_FUNCTIONS_e specialFunction)
This is the public function call access point to call dedicated special sub-functions.
volatile struct BUCK_LOOP_SETTINGS_s i_loop[BUCK_MPHASE_COUNT]
BUCK Current control loop objects.
volatile struct BUCK_CONVERTER_STATUS_s status
BUCK operation status bits.
#define CS_CALIB_STEPS
Number of signal oversampling steps used to determine the calibration value.
volatile uint16_t i_sns[BUCK_MPHASE_COUNT]
BUCK output current.
BUCK_SPECIAL_FUNCTIONS_e
Enumeration of special function sub-states.
#define BUCK_MPHASE_COUNT
Declaration of the number of power train phases of the Buck Converter.
volatile struct BUCK_CONVERTER_DATA_s data
BUCK runtime data.
volatile struct CS_CALIBRATION_s calib_cs[BUCK_MPHASE_COUNT]
Array of current sense calibration data objects of type CS_CALIBRATION_t.
volatile bool cs_calib_enable
Bit #8: Flag bit indicating that current sensors need to calibrated.
volatile struct BUCK_CONVERTER_SETTINGS_s set_values
Control field for global access to references.
volatile bool pwm_active
Bit #2: indicating that PWM has been started and ADC triggers are generated.
volatile bool adc_active
Bit #1: indicating that ADC has been started and samples are taken.
BUCK control & monitoring data structure.
volatile uint16_t CurrentSenseOffsetCalibration(volatile struct BUCK_CONVERTER_s *buckInstance)
Performs an offset calibration of the current sense feedback signal(s)
volatile uint16_t no_of_phases
number of converter phases
volatile uint16_t feedback_offset
Feedback offset value for calibration or bi-direction feedback signals.
BUCK_OPSTATE_RETURNS_e
Enumeration of state machine operating state return values.