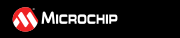 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
43 #ifndef BUCK_CONVERTER_STATE_MACHINE_H
44 #define BUCK_CONVERTER_STATE_MACHINE_H
50 #include "dev_buck_typedef.h"
volatile uint16_t drv_BuckConverter_Resume(volatile struct BUCK_CONVERTER_s *buckInstance)
This function resume the operation of the buck converter.
volatile uint16_t drv_BuckConverter_Start(volatile struct BUCK_CONVERTER_s *buckInstance)
This function starts the buck converter.
volatile uint16_t drv_BuckConverter_Initialize(volatile struct BUCK_CONVERTER_s *buckInstance)
This function initializes all peripheral modules and their instances used by the power controller.
volatile uint16_t drv_BuckConverter_Stop(volatile struct BUCK_CONVERTER_s *buckInstance)
This function stop the buck converter opration.
BUCK control & monitoring data structure.
volatile uint16_t drv_BuckConverter_Execute(volatile struct BUCK_CONVERTER_s *buckInstance)
This function is the main buck converter state machine executing the most recent state.
volatile uint16_t drv_BuckConverter_Suspend(volatile struct BUCK_CONVERTER_s *buckInstance)
This function suspends the operation of the buck converter.