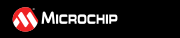 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
31 #ifndef APPLICATION_LAYER_POWER_CONTROL_H
32 #define APPLICATION_LAYER_POWER_CONTROL_H
39 #include "power_control/devices/dev_buck_typedef.h"
volatile uint16_t appPowerSupply_Resume(void)
This function resumes the power supply operation.
volatile uint16_t appPowerSupply_Stop(void)
This function calls the buck converter device driver function stopping the power supply.
volatile uint16_t appPowerSupply_Start(void)
This function calls the buck converter device driver function starting the power supply.
volatile uint16_t appPowerSupply_Suspend(void)
This function stops the power supply operation.
volatile uint16_t appPowerSupply_Execute(void)
This is the top-level function call triggering the most recent state machine of all associated power ...
BUCK control & monitoring data structure.
volatile uint16_t appPowerSupply_Initialize(void)
Calls the application layer power controller initialization.
volatile struct BUCK_CONVERTER_s buck
Global data object for a BUCK CONVERTER.