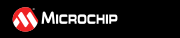 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
56 #ifndef _GLOBAL_TRAPS_H_
57 #define _GLOBAL_TRAPS_H_
73 #if ((__HAS_DMA__) || (__HAS_DMAV2__))
74 #define TRAP_DMA_SUPPORT 1
76 #define TRAP_DMA_SUPPORT 0
80 #define FAULT_OBJECT_CPU_RESET_TRIGGER_BIT_MASK 0b0000000000000001
81 #define CPU_RESET_TRIGGER_LOW_BIT_MASK 0b00000000000000011011101110000000
84 // =================================================================================================
101 TRAP_OSCILLATOR_FAIL = 0x0001,
102 TRAP_ADDRESS_ERROR = 0x0002,
103 TRAP_STACK_ERROR = 0x0004,
104 TRAP_MATH_ERROR = 0x0008,
105 TRAP_DMA_ERROR = 0x0010,
107 TRAP_SOFT_TRAP_ERROR = 0x0020,
108 TRAP_HARD_TRAP_ERROR = 0x0040,
109 TRAP_RESERVED_TRAP_5_ERROR = 0x0080,
110 TRAP_RESERVED_TRAP_7_ERROR = 0x0080,
112 TRAP_ALT_OSCILLATOR_FAIL = 0x0100,
113 TRAP_ALT_ADDRESS_ERROR = 0x0200,
114 TRAP_ALT_STACK_ERROR = 0x0400,
115 TRAP_ALT_MATH_ERROR = 0x0800,
116 TRAP_ALT_DMA_ERROR = 0x1000,
118 TRAP_ALT_SOFT_TRAP_ERROR = 0x2000,
119 TRAP_ALT_HARD_TRAP_ERROR = 0x4000,
121 TRAP_RESET_MSK = 0x7F1F
157 }__attribute__((packed))bits;
179 }__attribute__((packed))bits;
205 volatile unsigned cm :1;
213 }__attribute__((packed))bits;
250 }__attribute__((packed)) bits;
305 bool accumulator_a_overflow_trap_enable,
306 bool accumulator_b_overflow_trap_enable,
307 bool accumulator_catastrophic_overflow_trap_enable);
309 extern void __attribute__((__interrupt__)) _HardTrapError(
void);
310 extern void __attribute__((__interrupt__)) _SoftTrapError(
void);
312 extern void __attribute__((__interrupt__)) _OscillatorFail(
void);
313 extern void __attribute__((__interrupt__)) _AddressError(
void);
314 extern void __attribute__((__interrupt__)) _StackError(
void);
315 extern void __attribute__((__interrupt__)) _MathError(
void);
321 #if (TRAP_DMA_SUPPORT == 1)
322 #if defined (_DMACError)
323 extern void __attribute__((__interrupt__)) _DMACError(
void);
328 #if __XC16_VERSION__ < 1030
332 extern void __attribute__((interrupt, no_auto_psv)) _AltHardTrapError(
void);
333 extern void __attribute__((interrupt, no_auto_psv)) _AltSoftTrapError(
void);
335 extern void __attribute__((__interrupt__)) _AltOscillatorFail(
void);
336 extern void __attribute__((__interrupt__)) _AltAddressError(
void);
337 extern void __attribute__((__interrupt__)) _AltStackError(
void);
338 extern void __attribute__((__interrupt__)) _AltMathError(
void);
340 #if (TRAP_DMA_SUPPORT == 1)
341 extern void __attribute__((__interrupt__)) _AltDMACError(
void);
volatile unsigned OVBERR
Bit #1: Accumulator B Overflow Trap Flag bit.
enum TRAP_ID_e trap_id
Trap-ID of the captured incident.
struct CPU_INTTREG_s CPU_INTTREG_t
volatile unsigned CAN
Bit #17: CAN Address Error Soft Trap Status bit.
volatile struct TRAPLOG_STATUS_s status
Status word of the Trap Logger object.
Provides information for executed task, fault event and operating mode ID of task manager.
volatile unsigned
Bit #12: Reserved.
TRAP_ID_e
defining trap-ID for primary and secondary vectors
volatile unsigned SGHT
Bit #14: Software Generated Hard Trap Status bit.
volatile unsigned ADDRERR
Bit #7: Address Error Trap Status bit.
volatile unsigned trapr
Bit #15: Trap Reset Flag bit.
volatile unsigned swr
Bit #6: Software Reset Flag (Instruction) bit.
volatile unsigned sleep
Bit #3: Wake-up from Sleep Flag bit.
volatile unsigned NAE
Bit #11: NVM Address Error Soft Trap Status bit.
volatile unsigned extr
Bit #7: External Reset Pin (MCLR) bit.
volatile uint16_t trap_count
Counter tracking the number of occurrences.
volatile unsigned VHOLD
Bit #13: Vector Number Capture Enable bit.
volatile unsigned COVAERR
Bit #2: Accumulator A Catastrophic Overflow Trap Flag bit.
defining the CPU interrupt for primary and secondary vectors
volatile unsigned VECNUM
Bit #0-7: Pending Interrupt Number List.
volatile unsigned cm
Bit #9: Configuration Mismatch Flag bit.
volatile unsigned ILR
Bit #8-11: New Interrupt Priority Level.
Defines the Trap Flag for primary and secondary vectors.
volatile struct CPU_RCON_s rcon_reg
Captures the RESET CONTROL register.
Data structure for RCON status capturing.
volatile unsigned DMACERR
Bit #15: DMA Trap Status bit.
Data structure for RCON status capturing.
volatile unsigned COVBERR
Bit #3: Accumulator B Catastrophic Overflow Trap Flag bit.
volatile unsigned iopuwr
Bit #14: Illegal Opcode or Uninitialized W Access Reset Flag bit.
volatile unsigned APLL
Bit #13: Auxiliary PLL Loss of Lock Soft Trap Status bit.
volatile unsigned DIV0ERR
Bit #5: Divide-by-Zero Error Status bit.
struct TASK_INFO_s TASK_INFO_t
volatile uint16_t reset_count
Counter of CPU RESET events (read/write)
volatile unsigned ECCDBE
Bit #16: ECC Double-Bit Error Trap Status bit.
struct CPU_RCON_s CPU_RCON_t
volatile unsigned OSCFAIL
Bit #9: Oscillator Failure Trap Status bit.
volatile unsigned idle
Bit #2: Wake-up from Idle Flag bit.
volatile unsigned DOOVR
Bit #12: DO Stack Overflow Soft Trap Status bit.
volatile unsigned swdten
Bit #5: Software Enable/Disable of WDT bit.
volatile uint16_t fault_id
volatile unsigned SWTRAP
Bit #10: Software Trap Status bit.
volatile unsigned vregsf
Bit #11: Flash Voltage Regulator Standby During Sleep bit.
volatile struct TRAP_LOGGER_s traplog
data structure used as buffer for trap monitoring
struct TRAP_LOGGER_s TRAP_LOGGER_t
volatile unsigned vregs
Bit #8: Voltage Regulator Standby During Sleep bit.
volatile struct TASK_INFO_s task_capture
Information of last task executed.
volatile uint16_t task_id
volatile bool cpu_reset_trigger
volatile uint16_t op_mode
volatile uint16_t drv_TrapHandler_SoftTrapsInitialize(bool accumulator_a_overflow_trap_enable, bool accumulator_b_overflow_trap_enable, bool accumulator_catastrophic_overflow_trap_enable)
Configures the software-configurable traps.
struct TRAP_FLAGS_s TRAP_FLAGS_t
volatile unsigned
Bit <19:31> (reserved)
volatile unsigned wdto
Bit #4: Watchdog Timer Time-out Flag bit.
volatile unsigned OVAERR
Bit #0: Accumulator A Overflow Trap Flag bit.
Global data structure for trap event capturing.
struct TRAPLOG_STATUS_s TRAPLOG_STATUS_t
volatile struct CPU_INTTREG_s inttreg
Interrupt Vector and Priority register capture.
volatile unsigned CAN2
Bit #18: CAN2 Address Error Soft Trap Status bit.
volatile struct TRAP_FLAGS_s trap_flags
Complete list of trap flags (showing all trap flags)
volatile unsigned bor
Bit #1: Brown-out Reset Flag bit.
volatile unsigned STKERR
Bit #8: Stack Error Trap Status bit.
volatile unsigned MATHERR
Bit #6: Math Error Status bit.
volatile unsigned por
Bit #0: Power-on Reset Flag bit.
volatile unsigned
Bit #10: Reserved.
volatile unsigned SFTACERR
Bit #4: Shift Accumulator Error Status bit.