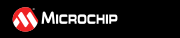 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
44 #include "drv_fault_handler.h"
59 .Status.bits.Enabled =
false,
60 .Status.bits.FaultActive =
true,
61 .Status.bits.FaultStatus =
true,
63 .SourceObject.ptrObject = NULL,
64 .SourceObject.bitMask = 0xFFFF,
65 .ReferenceObject.ptrObject = NULL,
66 .ReferenceObject.bitMask = 0xFFFF,
67 .TripResponse.compareThreshold = 0,
68 .TripResponse.eventThreshold = 0,
69 .TripResponse.ptrResponseFunction = NULL,
70 .RecoveryResponse.compareThreshold = 0,
71 .RecoveryResponse.eventThreshold = 0,
72 .RecoveryResponse.ptrResponseFunction = NULL,
170 volatile uint16_t retval=1;
171 volatile uint16_t source=0;
174 if (fltObject == NULL)
189 source = (
volatile uint16_t)
190 abs((
volatile int32_t)source - (
volatile int32_t)reference);
197 case FLTCMP_GREATER_THAN:
205 case FLTCMP_LESS_THAN:
212 case FLTCMP_IS_EQUAL:
219 case FLTCMP_IS_NOT_EQUAL:
enum FLT_COMPARE_TYPE_e CompareType
Bit <10:8>: Fault check comparison type control bits.
volatile struct FLT_EVENT_RESPONSE_s RecoveryResponse
Settings defining the fault recovery event.
volatile struct FLT_OBJECT_STATUS_s Status
Status word of this fault object.
volatile struct FLT_EVENT_RESPONSE_s TripResponse
Settings defining the fault trip event.
volatile bool FaultActive
Bit 1: Flag bit indicating if fault condition has been detected but FAULT has not been tripped yet.
volatile struct FAULT_OBJECT_s fltObjectClear
Clears the fault objects.
volatile struct FLT_COMPARE_OBJECT_s SourceObject
Object which should be monitored.
volatile struct FLT_COMPARE_OBJECT_s ReferenceObject
Reference object the source should be compared with.
volatile uint16_t eventThreshold
Bit mask will be &-ed with source as value (use 0xFFFF for full value comparison)
volatile uint16_t compareThreshold
Signal level at which the fault condition will be detected.
volatile bool Enabled
Bit 15: Control bit enabling/disabling monitoring of the fault object.
volatile uint16_t drv_FaultHandler_CheckObject(volatile struct FAULT_OBJECT_s *fltObject)
Check current fault status of a user-defined fault object.
volatile uint16_t * ptrObject
Pointer to register or variable which should be monitored.
volatile uint16_t(* ptrResponseFunction)(void)
pointer to a user-defined function called when a defined fault monitoring event is detected
volatile uint16_t Counter
Fault event counter (controlled by FAULT HANDLER)
This data structure is a collection of data structures for fault handling.
volatile uint16_t bitMask
Bit mask will be &-ed with source as value (use 0xFFFF for full value comparison)
volatile bool FaultStatus
Bit 0: Flag bit indicating if FAULT has been tripped.