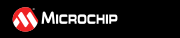 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
77 __builtin_write_RPCON(0x0800);
78 return(RPCONbits.IOLOCK);
104 __builtin_write_RPCON(0x0000);
105 return(1 - RPCONbits.IOLOCK);
133 volatile uint16_t
PPS_RemapOutput(
volatile uint8_t pinno,
volatile uint8_t peripheral){
135 volatile uint16_t retval = 0;
136 volatile uint8_t *regptr;
137 volatile uint8_t pin_offset=0;
139 pin_offset = (pinno - RP_PINNO_MIN);
140 regptr = (
volatile uint8_t *)&RPOR0;
141 regptr += (
volatile uint8_t)pin_offset;
142 *regptr = (
volatile uint8_t)peripheral;
143 retval = (bool)(*regptr == (
volatile uint8_t)peripheral);
174 volatile uint16_t
PPS_RemapInput(
volatile uint8_t pinno,
volatile uint8_t *peripheral)
209 volatile uint16_t retval=0;
242 volatile uint16_t retval=0;
volatile uint16_t PPS_UnmapInput(volatile uint8_t *peripheral)
Disconnects a pin from a digital function input.
volatile uint16_t PPS_LockIO(void)
Locks the Peripheral Pin Select Configuration registers against accidental changes.
volatile uint16_t PPS_UnlockIO(void)
Unlocks the Peripheral Pin Select Configuration registers to enable changes.
volatile uint16_t PPS_RemapInput(volatile uint8_t pinno, volatile uint8_t *peripheral)
Assigns a pin to a digital function input.
volatile uint16_t PPS_RemapOutput(volatile uint8_t pinno, volatile uint8_t peripheral)
Assigns a digital function output to a pin.
volatile uint16_t PPS_UnmapOutput(volatile uint8_t pinno)
Disconnects a pin from a digital function output.