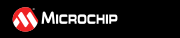 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
35 #ifndef P33C_DAC_SFR_ABSTRACTION_H
36 #define P33C_DAC_SFR_ABSTRACTION_H
46 #ifndef P33C_DAC_MODULE_s
62 volatile struct tagDACCTRL1LBITS
bits;
67 volatile struct tagDACCTRL2LBITS
bits;
71 volatile struct tagDACCTRL2HBITS
bits;
72 volatile uint16_t
value;
74 } __attribute__((packed));
80 #ifndef P33C_DAC_INSTANCE_s
96 volatile struct tagDAC1CONLBITS
bits;
100 volatile struct tagDAC1CONHBITS
bits;
104 volatile struct tagDAC1DATLBITS
bits;
108 volatile struct tagDAC1DATHBITS
bits;
112 volatile struct tagSLP1CONLBITS
bits;
116 volatile struct tagSLP1CONHBITS
bits;
120 volatile struct tagSLP1DATBITS
bits;
121 volatile uint16_t
value;
123 } __attribute__((packed));
135 #define P33C_DAC_SFR_OFFSET ((volatile uint16_t)&DAC2CONL - (volatile uint16_t)&DAC1CONL)
155 volatile uint16_t dacInstance
159 volatile uint16_t dacInstance
163 volatile uint16_t dacInstance
167 volatile uint16_t dacInstance,
volatile struct P33C_DAC_INSTANCE_s * p33c_DacInstance_GetHandle(volatile uint16_t dacInstance)
Gets pointer to DAC Instance SFR set.
volatile uint16_t p33c_DacInstance_Dispose(volatile uint16_t dacInstance)
Resets all DAC Instance registers to their RESET default values.
volatile struct tagDAC1CONLBITS bits
union P33C_DAC_MODULE_s::@0 DacModuleCtrl1L
volatile struct P33C_DAC_INSTANCE_s dacConfigClear
Default RESET configuration of one DAC instance channel SFRs.
volatile struct P33C_DAC_MODULE_s * p33c_DacModule_GetHandle(void)
Gets pointer to DAC Module SFR set.
union P33C_DAC_INSTANCE_s::@11 SLPxCONH
volatile uint16_t p33c_DacInstance_ConfigWrite(volatile uint16_t dacInstance, volatile struct P33C_DAC_INSTANCE_s dacConfig)
Writes a user-defined configuration to the DAC instance registers.
volatile struct P33C_DAC_MODULE_s dacModuleDefault
Default configuration of DAC module running from 500 MHz input clock.
union P33C_DAC_INSTANCE_s::@8 DACxDATL
volatile uint16_t p33c_DacModule_ConfigWrite(volatile struct P33C_DAC_MODULE_s dacConfig)
Writes a user-defined configuration to the DAC module base registers.
union P33C_DAC_INSTANCE_s::@7 DACxCONH
volatile struct P33C_DAC_MODULE_s p33c_DacModule_ConfigRead(void)
Read the current configuration from the DAC module base registers.
Abstracted set of Special Function Registers of a Digital-to-Analog Converter peripheral.
volatile struct tagDACCTRL1LBITS bits
union P33C_DAC_INSTANCE_s::@12 SLPxDAT
union P33C_DAC_MODULE_s::@1 DacModuleCtrl2L
union P33C_DAC_INSTANCE_s::@9 DACxDATH
volatile struct P33C_DAC_MODULE_s dacModuleConfigClear
Default RESET configuration of the DAC module base SFRs.
volatile uint16_t p33c_DacModule_Dispose(void)
Resets all DAC Module registers to their RESET default values.
union P33C_DAC_INSTANCE_s::@6 DACxCONL
union P33C_DAC_MODULE_s::@2 DacModuleCtrl2H
union P33C_DAC_INSTANCE_s::@10 SLPxCONL
volatile struct P33C_DAC_INSTANCE_s p33c_DacInstance_ConfigRead(volatile uint16_t dacInstance)
Read the current configuration from the DAC instance registers.