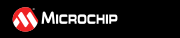 |
Digital Power Starter Kit 3 Firmware
dsPIC33C Buck Converter Voltage Mode Control Example
|
|
34 .CCPxCON1L.value = 0x0000,
66 ((
volatile uint8_t*)&CCP1CON1L + ((ccpInstance - 1) * P33C_CCPGEN_SFR_OFFSET));
92 ((
volatile uint8_t*)&CCP1CON1L + ((ccpInstance - 1) * P33C_CCPGEN_SFR_OFFSET));
119 volatile uint16_t retval=1;
124 ((
volatile uint8_t*)&CCP1CON1L + ((ccpInstance - 1) * P33C_CCPGEN_SFR_OFFSET));
Generic Capture Compare Driver Module (header file)
volatile struct P33C_CCP_INSTANCE_SFRSET_s ccpConfigDefault
CCP Register Set reset state template.
volatile struct P33C_CCP_INSTANCE_SFRSET_s p33c_CcpInstance_ConfigRead(volatile uint16_t ccpInstance)
Read the current configuration from the CCP instance registers.
volatile struct P33C_CCP_INSTANCE_SFRSET_s * p33c_CcpInstance_GetHandle(volatile uint16_t ccpInstance)
Gets pointer to CCP instance SFR set.
union P33C_CCP_INSTANCE_SFRSET_s::@6 CCPxTMRL
volatile uint16_t p33c_CcpInstance_ConfigWrite(volatile uint16_t ccpInstance, volatile struct P33C_CCP_INSTANCE_SFRSET_s ccpConfig)
Writes a user-defined configuration to the CCP instance registers.